Handling Out Of Range Errors: Difference between revisions
No edit summary |
(→Intro) |
||
Line 2: | Line 2: | ||
==Intro== | ==Intro== | ||
All new Phidgets have been designed to seamlessly and informatively communicate problems with measured data. This allows you to more easily debug your systems, while ensuring data events from sensors continue at a steady pace. This makes it possible to use data events as a reliable time-base for your system. | All new Phidgets have been designed to seamlessly and informatively communicate problems with measured data. This allows you to more easily debug your systems, while ensuring data events from sensors continue at a steady pace. This makes it possible to use data events as a reliable time-base for your system. | ||
<center>[[Image:OutOfRangeErrorSampleGraph.png|400px|Center|link=|]]</center> | |||
To understand the more technical points of this article, we recommend first learning about [[Phidget Programming Basics]]. | To understand the more technical points of this article, we recommend first learning about [[Phidget Programming Basics]]. |
Revision as of 16:59, 14 July 2023
Intro
All new Phidgets have been designed to seamlessly and informatively communicate problems with measured data. This allows you to more easily debug your systems, while ensuring data events from sensors continue at a steady pace. This makes it possible to use data events as a reliable time-base for your system.
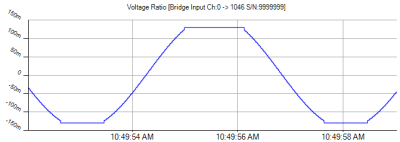
To understand the more technical points of this article, we recommend first learning about Phidget Programming Basics.
Data Events
All modern Phidgets guarantee that data events will occur on a channel at the user-defined rate, or will inform you of the actual data rate if it is unable to keep up. This remains true even if the data on the channel is unknown or invalid.
Values from a sensor that are too high or too low to be accurately measured will be capped slightly outside the specified Minimum and Maximum values for the sensor. For example, if a temperature sensor can measure from -30°C to 85°C, you can expect values outside of this range to all read as -31°C and 86°C, respectively. You can monitor for these out-of-range indicators by checking the value from the event against the minimum and maximum values for the sensor.
Values that are strictly unknown for one reason or another, such as a probe being unplugged, will be reported as NaN in the related event handler. Be sure your code is robust enough to handle this possibility.
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
import math
import time
def onTemperatureChange(self, temperature):
if(math.isnan(temperature)):
print("Temperature is unknown")
elif(temperature > self.getMaxTemperature()):
print("Temperature is too high")
elif(temperature < self.getMinTemperature()):
print("Temperature is too low")
else:
print("Temperature: " + str(temperature))
def main():
temperatureSensor0 = TemperatureSensor()
temperatureSensor0.setOnTemperatureChangeHandler(onTemperatureChange)
temperatureSensor0.openWaitForAttachment(5000)
try:
input("Press Enter to Stop\n")
except (Exception, KeyboardInterrupt):
pass
temperatureSensor0.close()
main()
import com.phidget22.*;
import java.util.Scanner; //Required for Text Input
import java.io.IOException;
public class Java_Example {
public static void main(String[] args) throws Exception {
TemperatureSensor temperatureSensor0 = new TemperatureSensor();
temperatureSensor0.addTemperatureChangeListener(new
TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
try {
if(Double.isNaN(e.getTemperature()))
System.out.println("Temperature is unknown");
else if(e.getTemperature() > temperatureSensor0.getMaxTemperature())
System.out.println("Temperature is too high");
else if(e.getTemperature() < temperatureSensor0.getMinTemperature())
System.out.println("Temperature is too low");
else
System.out.println("Temperature: " + e.getTemperature());
} catch (PhidgetException ex) {
System.out.println("PhidgetException " +
ex.getErrorCode() + " (" + ex.getDescription() + "): " +
ex.getDetail());
}
}
});
temperatureSensor0.open(5000);
//Wait until Enter has been pressed before exiting
System.in.read();
temperatureSensor0.close();
}
}
using System;
using Phidget22;
namespace ConsoleApplication
{
class Program
{
private static void TemperatureSensor0_TemperatureChange(object sender, Phidget22.Events.TemperatureSensorTemperatureChangeEventArgs e)
{
TemperatureSensor ch = (TemperatureSensor)sender;
if (Double.IsNaN(e.Temperature))
Console.WriteLine("Temperature is unknown");
else if (e.Temperature > ch.MaxTemperature)
Console.WriteLine("Temperature is too high");
else if (e.Temperature < ch.MinTemperature)
Console.WriteLine("Temperature is too low");
else
Console.WriteLine("Temperature: " + e.Temperature);
}
static void Main(string[] args)
{
TemperatureSensor temperatureSensor0 = new TemperatureSensor();
temperatureSensor0.TemperatureChange += TemperatureSensor0_TemperatureChange;
temperatureSensor0.Open(5000);
//Wait until Enter has been pressed before exiting
Console.ReadLine();
temperatureSensor0.Close();
}
}
}
Press Enter to Stop
Temperature: 14.062
Temperature: 14.056
Temperature: 14.16
Temperature: 389.105
Temperature is too high
Temperature is too high
Temperature is too high
Temperature: 504.21
Temperature: -198.721
Temperature is too low
Temperature is too low
Temperature is too low
Temperature is too low
Temperature: -153.69
Temperature: 19.306
Temperature: 17.559
Temperature: 17.637
Temperature: 17.629
Temperature is unknown
Temperature is unknown
Temperature is unknown
Temperature is unknown
Temperature: 15.55
Temperature: 16.549
Temperature: 16.978
Temperature: 17.214
Polling
When polling for data, values that are unknown, too high, or too low will give relevant return codes or exceptions, typically UNKNOWNVALUE, UNKNOWNVALUEHIGH, or UNKNOWNVALUELOW respectively.
In most programming languages (excluding C), specific information about the error will be available to the exception handler to help identify the specifics of the error.
For information on how to see error messages in C, look up Phidget_getLastError in the API.
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
import math
import time
def main():
temperatureSensor0 = TemperatureSensor()
temperatureSensor0.openWaitForAttachment(5000)
for i in range(1, 10):
try:
print(temperatureSensor0.getTemperature())
except PhidgetException as ex:
print("PhidgetException " + str(ex.code) + " (" + ex.description + "): " + ex.details)
time.sleep(1)
temperatureSensor0.close()
main()
import com.phidget22.*;
import java.util.Scanner; //Required for Text Input
import java.io.IOException;
public class Java_Example {
public static void main(String[] args) throws Exception {
TemperatureSensor temperatureSensor0 = new TemperatureSensor();
temperatureSensor0.open(5000);
while(System.in.available() == 0) {
try {
System.out.println(temperatureSensor0.getTemperature());
} catch (PhidgetException ex) {
System.out.println("PhidgetException " +
ex.getErrorCode() + " (" + ex.getDescription() + "): " +
ex.getDetail());
}
Thread.sleep(1000);
}
temperatureSensor0.close();
}
}
using System;
using Phidget22;
namespace ConsoleApplication
{
class Program
{
static void Main(string[] args)
{
TemperatureSensor temperatureSensor0 = new TemperatureSensor();
temperatureSensor0.Open(5000);
while(Console.KeyAvailable == false)
{
try
{
Console.WriteLine("Temperature: " + temperatureSensor0.Temperature);
}
catch (PhidgetException ex)
{
Console.WriteLine("PhidgetException " + ex.ErrorCode +
" (" + ex.Description + "): " + ex.Detail);
}
System.Threading.Thread.Sleep(1000);
}
temperatureSensor0.Close();
}
}
}
24.041
24.052
PhidgetException 60 (Invalid Value - Too High): The value has been measured to be higher than the valid range of the sensor.
PhidgetException 60 (Invalid Value - Too High): The value has been measured to be higher than the valid range of the sensor.
222.166
-132.291
PhidgetException 61 (Invalid Value - Too Low): The value has been measured to be lower than the valid range of the sensor.
PhidgetException 61 (Invalid Value - Too Low): The value has been measured to be lower than the valid range of the sensor.
-132.632
119.862
120.613
PhidgetException 51 (Unknown or Invalid Value): The value is unknown. This can happen right after attach, when the value has not yet been received from the Phidget. This can also happen if a device has not yet been configured / enabled. Some properties can only be read back after being set.
PhidgetException 51 (Unknown or Invalid Value): The value is unknown. This can happen right after attach, when the value has not yet been received from the Phidget. This can also happen if a device has not yet been configured / enabled. Some properties can only be read back after being set.
120.045
Error Events
In addition to data events, you can use error event handlers to get specific information about error conditions as they happen. A single error event handler will provide meaningful information in text form about each error condition as it happens. These have been standardized to occur once every second for as long as the error condition persists.
If your system has older Phidgets, this method may be the only way to access additional error information.
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
import time
def onError(self, code, description):
print("Code: " + ErrorEventCode.getName(code))
print("Description: " + str(description))
print("----------")
def main():
temperatureSensor0 = TemperatureSensor()
temperatureSensor0.setOnErrorHandler(onError)
temperatureSensor0.openWaitForAttachment(5000)
try:
input("Press Enter to Stop\n")
except (Exception, KeyboardInterrupt):
pass
temperatureSensor0.close()
main()
import com.phidget22.*;
import java.lang.InterruptedException;
public class Java_Example {
public static void main(String[] args) throws Exception {
TemperatureSensor temperatureSensor0 = new TemperatureSensor();
temperatureSensor0.addErrorListener(new ErrorListener() {
public void onError(ErrorEvent e) {
System.out.println("Code: " + e.getCode().name());
System.out.println("Description: " + e.getDescription());
System.out.println("----------");
}
});
temperatureSensor0.open(5000);
//Wait until Enter has been pressed before exiting
System.in.read();
temperatureSensor0.close();
}
}
using System;
using Phidget22;
namespace ConsoleApplication
{
class Program
{
private static void TemperatureSensor0_Error(object sender, Phidget22.Events.ErrorEventArgs e)
{
Console.WriteLine("Code: " + e.Code);
Console.WriteLine("Description: " + e.Description);
Console.WriteLine("----------");
}
static void Main(string[] args)
{
TemperatureSensor temperatureSensor0 = new TemperatureSensor();
temperatureSensor0.Error += TemperatureSensor0_Error;
temperatureSensor0.Open(5000);
//Wait until Enter has been pressed before exiting
Console.ReadLine();
temperatureSensor0.Close();
}
}
}
Press Enter to Stop
Code: EEPHIDGET_OUTOFRANGEHIGH
Description: Temperature is too high to be accurately measured
----------
Code: EEPHIDGET_OUTOFRANGEHIGH
Description: Temperature is too high to be accurately measured
----------
Code: EEPHIDGET_OUTOFRANGELOW
Description: Temperature is too low to be accurately measured
----------
Code: EEPHIDGET_OUTOFRANGELOW
Description: Temperature is too low to be accurately measured
----------
Code: EEPHIDGET_BADCONNECTION
Description: Bad Connection: RTD is likely disconnected
----------
Code: EEPHIDGET_BADCONNECTION
Description: Bad Connection: RTD is likely disconnected
----------