What is a Phidget?: Difference between revisions
No edit summary |
No edit summary |
||
Line 2: | Line 2: | ||
==Introduction== | ==Introduction== | ||
Phidgets are building- | Phidgets are building-blocks for sensing and control using a computer, tablet, or phone. Users create applications that use Phidgets to interact with the physical world. Phidgets connect via a USB port: | ||
[[Image:wiap-image1.jpg|500px|link=|alt=|center]] | [[Image:wiap-image1.jpg|500px|link=|alt=|center]] | ||
Line 8: | Line 8: | ||
---- | ---- | ||
Some Phidgets are a complete, self-contained sensing package. An example is then [{{SERVER}}/products.php?product_id=1042 1042] | |||
Some Phidgets are a complete, self-contained sensing package. | |||
, which measures motion: | , which measures motion: | ||
[[Image:wiap-image2-spatial.jpg|500px|link=|alt=|center]] | [[Image:wiap-image2-spatial.jpg|500px|link=|alt=|center]] | ||
Line 16: | Line 15: | ||
---- | ---- | ||
Other Phidgets are a 'building block' to use other sensors. An example is the [{{SERVER}}/products.php?product_id=1048 1048] which allows the use of wire thermocouples: | |||
[[Image:wiap-image2-temp.jpg|700px|link=|alt=|center]] | [[Image:wiap-image2-temp.jpg|700px|link=|alt=|center]] | ||
Line 23: | Line 21: | ||
---- | ---- | ||
Another Phidget may be a flexible I/O board which can receive analog sensor data and control digital inputs and outputs. An example is the [{{SERVER}}/products.php?product_id=1018 1018], with eight ports of each type: | |||
[[Image:wiap-image2-ifkt.jpg|700px|link=|alt=|center]] | [[Image:wiap-image2-ifkt.jpg|700px|link=|alt=|center]] | ||
---- | ---- | ||
Finally, a Phidget may be a [{{SERVER}}/products.php?product_id=HUB0000 VINT Hub], made up of versatile ports that can be used as inputs or outputs, and also connect to smart [[What_is_VINT?|VINT]] devices. | |||
[[Image:wiap-vint.jpg|700px|link=|alt=|center]] | [[Image:wiap-vint.jpg|700px|link=|alt=|center]] | ||
==Data Flow== | |||
Data and control flows up and down the USB connection: | Data and control flows up and down the USB connection: | ||
[[Image:wiap-dataflow.jpg|700px|link=|alt=|center]] | [[Image:wiap-dataflow.jpg|700px|link=|alt=|center]] | ||
== Software Channels == | |||
A Phidget device exports one or more channels. Each device channel implements a channel class where that class defines the interface used to access the features of the device in a generic fashion. For example, a Phidget device that supports sensing voltage exports a channel that implements the VoltageInput class. The VoltageInput class defines the methods, properties, events and errors relevant to receiving voltage data. | |||
[[Image:voltageInput_object.jpg|300px|link=|alt=|center]] | [[Image:voltageInput_object.jpg|300px|link=|alt=|center]] | ||
You can check | You can check the {{Phidget22API}} for details about channel classes. For example, the [{{SERVER}}/products.php?product_id=1018 1018] has five channels (with five distinct channel classes): | ||
[[Image:1018_objects.jpg|600px|link=|alt=|center]] | [[Image:1018_objects.jpg|600px|link=|alt=|center]] | ||
Each Phidget channel class inherits common functionality from the base Phidget class. The Phidget class defines methods like {{Code|open()}} and {{Code|close()}}, and properties like {{Code|DeviceSerialNumber}}. | |||
To help understand the concepts above, we have included a simple C# program that can be used with the [{{SERVER}}/products.php?product_id=1018 1018]. | |||
To help | |||
<syntaxhighlight lang=csharp> | <syntaxhighlight lang=csharp> | ||
Line 66: | Line 53: | ||
int main() { | int main() { | ||
VoltageInput vin; // | VoltageInput vin; // A user channel of the class VoltageInput | ||
vin.Open(5000); // | vin = new VoltageInput(); // Create an instance of the class | ||
vin.Channel = 0; // Specify the index of the device channel to attach to | |||
vin.Open(5000); // Begin attempting to attach to the device channel | |||
System.Console.WriteLine("My channel 0 sensor reads " + vin.Voltage); // Get the voltage value | System.Console.WriteLine("My channel 0 sensor reads " + vin.Voltage); // Get the voltage value | ||
vin.Close(); // | vin.Close(); // Close the channel, which detached it from the device channel | ||
} | } | ||
</syntaxhighlight> | </syntaxhighlight> | ||
Line 82: | Line 67: | ||
==Programming== | ==Programming== | ||
For the most part, using Phidgets requires writing software. The {{Phidget22API}} is available in many different programming languages: | |||
{| style="border:1px solid darkgray;" cellpadding="5px;" | {| style="border:1px solid darkgray;" cellpadding="5px;" | ||
Line 105: | Line 91: | ||
The Phidget software libraries are supported on a number of operating systems: | |||
{| style="border:1px solid darkgray;" cellpadding="7px;" | {| style="border:1px solid darkgray;" cellpadding="7px;" | ||
|-style="background: #f0f0f0" align=center | |-style="background: #f0f0f0" align=center |
Revision as of 16:22, 11 July 2017
Introduction
Phidgets are building-blocks for sensing and control using a computer, tablet, or phone. Users create applications that use Phidgets to interact with the physical world. Phidgets connect via a USB port:
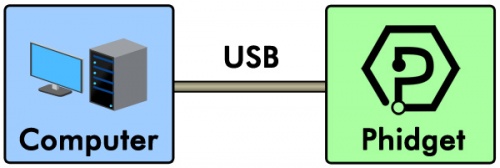
Some Phidgets are a complete, self-contained sensing package. An example is then 1042 , which measures motion:
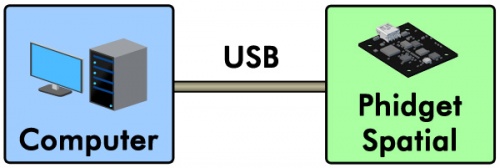
Other Phidgets are a 'building block' to use other sensors. An example is the 1048 which allows the use of wire thermocouples:
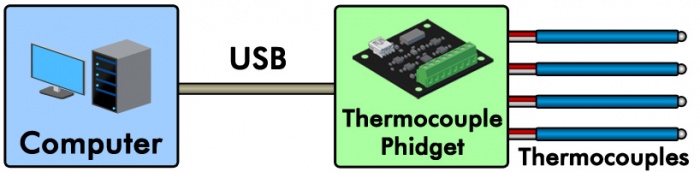
Another Phidget may be a flexible I/O board which can receive analog sensor data and control digital inputs and outputs. An example is the 1018, with eight ports of each type:
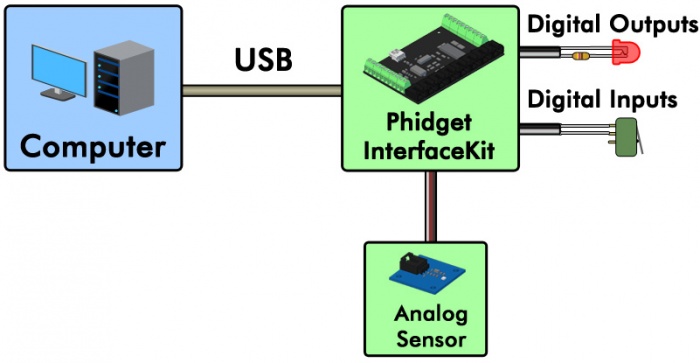
Finally, a Phidget may be a VINT Hub, made up of versatile ports that can be used as inputs or outputs, and also connect to smart VINT devices.
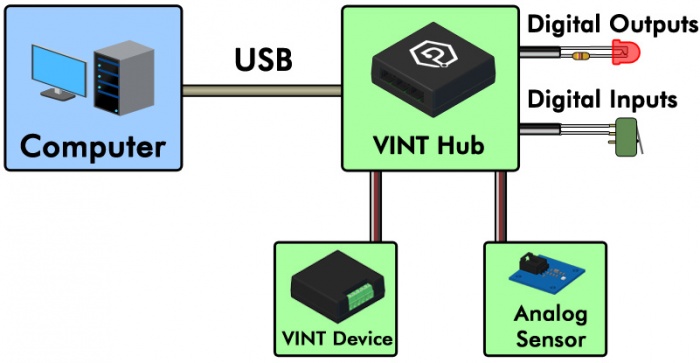
Data Flow
Data and control flows up and down the USB connection:
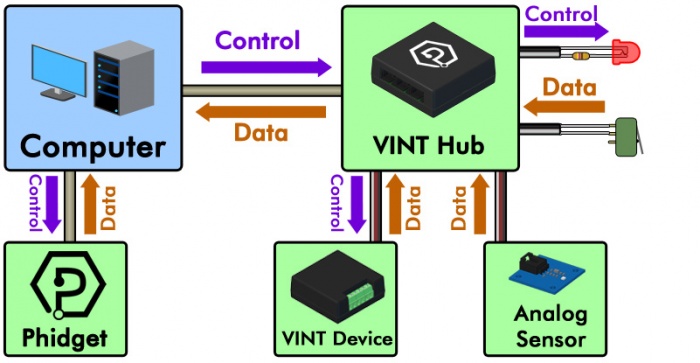
Software Channels
A Phidget device exports one or more channels. Each device channel implements a channel class where that class defines the interface used to access the features of the device in a generic fashion. For example, a Phidget device that supports sensing voltage exports a channel that implements the VoltageInput class. The VoltageInput class defines the methods, properties, events and errors relevant to receiving voltage data.
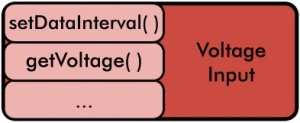
You can check the Phidget22 API for details about channel classes. For example, the 1018 has five channels (with five distinct channel classes):
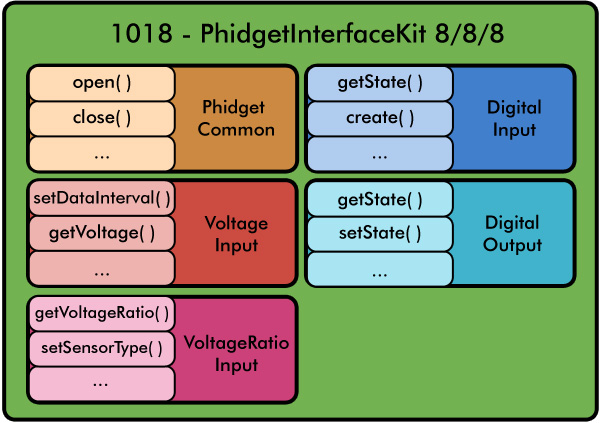
Each Phidget channel class inherits common functionality from the base Phidget class. The Phidget class defines methods like open()
and close()
, and properties like DeviceSerialNumber
.
To help understand the concepts above, we have included a simple C# program that can be used with the 1018.
using Phidget22;
int main() {
VoltageInput vin; // A user channel of the class VoltageInput
vin = new VoltageInput(); // Create an instance of the class
vin.Channel = 0; // Specify the index of the device channel to attach to
vin.Open(5000); // Begin attempting to attach to the device channel
System.Console.WriteLine("My channel 0 sensor reads " + vin.Voltage); // Get the voltage value
vin.Close(); // Close the channel, which detached it from the device channel
}
Programming
For the most part, using Phidgets requires writing software. The Phidget22 API is available in many different programming languages:
Core Languages | Mobile Languages | Other Languages |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() | ||
![]() | ||
![]() | ||
![]() |
The Phidget software libraries are supported on a number of operating systems:
Desktop OSes | Mobile/Wireless OSes |
![]() |
![]() |
![]() |
![]() |
![]() |
Network Server
Not only can you control a Phidget locally, but we also provide a tool called the Phidget Network Server. The Network Server exports your Phidget's channels over your local network:
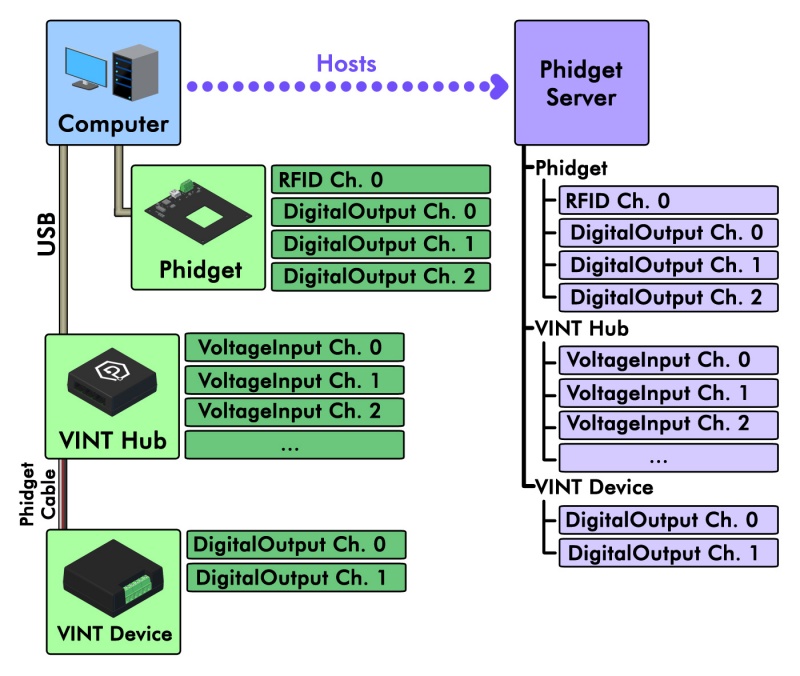
This allows other computers on your network to control the Phidget or read data from it. The network server isn't limited to desktop computers- it can also be used with phones or Single Board Computers that are running Phidgets code.
The Network Server also includes the Phidget Dictionary, which is a central place to store your data in key-value pairs.
Further Reading
With the combination of events, modular sensors, and network support, your system can range from simple to incredibly complex.
We encourage customers to not only build projects for themselves, but also to design and build real-world products using Phidgets. Our libraries can be distributed with your code to your customers.
And this can all occur with the same devices, and the same flexible software API.
Want to learn more? Check out our:
- Products on our main website
- Languages and Operating Systems
- Example Projects and Articles
- In-depth hardware information
Questions? Please contact us.