Prerequisites
This project assumes you are familiar with the following
- Arrays and/or lists.
- Sorting arrays and/or lists.
Setup
VINT Hub
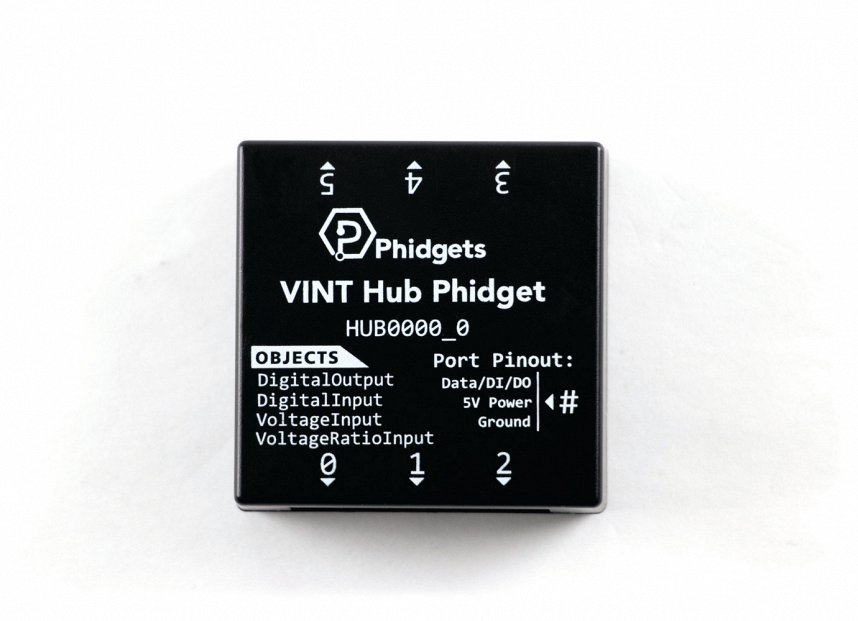
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
import com.phidget22.*;
public class WhistleDetector {
public static double max(double[] vals){
double max = vals[0];
for(int i = 1; i < vals.length; i++){
if(vals[i] > max){
max = vals[i];
}
}
return max;
}
public static int indexof(double val, double[] arr){
for(int i = 0; i < arr.length; i++){
if(val == arr[i]){
return i;
}
}
return 0;
}
public static void main(String[] args) throws Exception{
//Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
//Retrieve frequency band information
double[] octaves = soundSensor.getOctaves();
//Determine the dominate frequency, and return the index
int max_index = indexof(max(octaves), octaves);
System.out.println("Frequency: " + octaves_val[max_index] + " Hz");
Thread.sleep(250);
}
}
}
package whistledetector;
//Add Phidgets Library
import com.phidget22.*;
public class WhistleDetector {
public static double max(double[] vals){
double max = vals[0];
for(int i = 1; i < vals.length; i++){
if(vals[i] > max){
max = vals[i];
}
}
return max;
}
public static int indexof(double val, double[] arr){
for(int i = 0; i < arr.length; i++){
if(val == arr[i]){
return i;
}
}
return 0;
}
public static void main(String[] args) throws Exception{
//Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
//Retrieve frequency band information
double[] octaves = soundSensor.getOctaves();
//Determine the dominate frequency, and return the index
int max_index = indexof(max(octaves), octaves);
System.out.println("Frequency: " + octaves_val[max_index] + " Hz");
Thread.sleep(250);
}
}
}
Code not available.
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.SoundSensor import *
#Required for sleep statement
import time
#Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
octaves_val = [31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000]
#Create
soundSensor = SoundSensor()
#Open
soundSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
#Retrieve frequency band information
octaves = soundSensor.getOctaves()
#Determine the dominant frequency, and return the index
max_index = octaves.index(max(octaves))
#Print the corresponding frequency
print("Frequency: " + str(octaves_val[max_index]) + " Hz")
time.sleep(0.25)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using Phidget22;
namespace WhistleDetector
{
internal class Program
{
static void Main(string[] args)
{
//Define | The Sound Phidget can reports information about 10 indiviual frequency bands.
double[] octaves_val = {31.5, 63, 125, 250, 500, 1000, 2000, 4000, 8000, 16000};
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.Open(1000);
//Use your Phidgets
while (true)
{
//Retrieve frequency band information
double[] octaves = soundSensor.Octaves;
//Determine the dominate frequency, and return the index
int max_index = octaves.ToList().IndexOf(octaves.Max());
System.Console.WriteLine("Frequency: " + octaves_val[max_index] + " Hz");
System.Threading.Thread.Sleep(250);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
Code not available.
Run Your Program
Run your program and then whistle into the sensor. You will see the frequency of your whistle reported.
Code Review
The Sound Phidget returns information about 10 different frequency bands (31.5Hz, 63Hz, 125Hz, 250Hz, 500Hz, 1kHz, 2kHz, 4kHz, 8kHz, and 16kHz). In the code above, we identify the loudest band, and return the frequency value to the user.
Practice
- After you have identified the frequency of your whistle (it should be around 1000 Hz), modify your program to print Whistle Detected when you whistle into the sensor. Hint: if your whistle is 1000Hz, you should identify when that is the loudest of all bands (the maximum) and you should set a threshold so a whistle is only detected if the sound is louder than the ambient environment.
- Now that you can identify your whistle, try turning on the LEDs from the Getting Started Kit when your whistle is detected. Next, try creating a custom program that performs a function when your whistle is detected (e.g. add a Power Plug Phidget to turn lights on/off).
- Bonus: The Sound Phidget can detect the following frequencies: 31.5Hz, 63Hz, 125Hz, 250Hz, 500Hz, 1kHz, 2kHz, 4kHz, 8kHz, 16kHz. Try using an online tone generator to play sounds from your speaker. See if the Sound Phidget can detect each frequency band accurately.