Theremin
A theremin is a unique instrument that is completely contactless. Instead of musicians handling the instrument, they simply move their hands in the air above. The position of the musician's hands determines the sound produced. Check out this video for an example.
In this project, you will recreate this futuristic, non-contact effect using a Distance Phidget.
Setup
Before getting started, make sure you have the following parts.
Note: you can also use a Sonar Phidget, or any distance sensing Phidget for this project.
VINT Hub
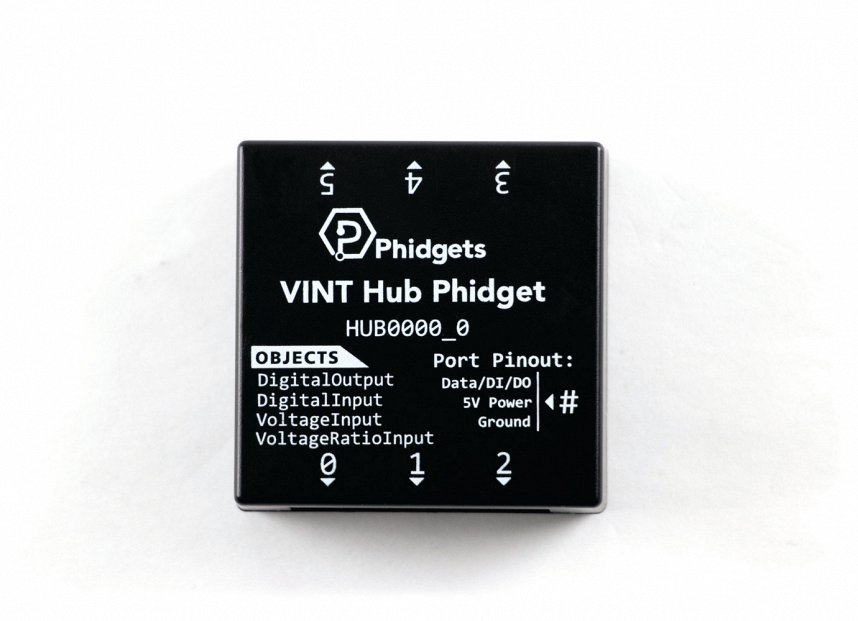
Write code (Java)
Copy the code below into a new Processing project. If you need a reminder of how to do this, revisit the Getting Started Course.
//Add Phidgets Library
import com.phidget22.*;
//Add Sound library
import processing.sound.*;
//Define
DistanceSensor pitch;
SinOsc sine;
void setup(){
try{
//Create
pitch = new DistanceSensor();
sine = new SinOsc(this);
sine.play();
//Open
pitch.open(1000);
//Configure
pitch.setDataInterval(pitch.getMinDataInterval());
}catch(Exception e){
//Handle Exceptions
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Freq Distance: " + pitch.getDistance());
//Map distance to frequency
float freqResult = map(pitch.getDistance(), 0, pitch.getMaxDistance(),20.0,1000.0);
sine.freq(freqResult);
delay(30);
}catch(Exception e){
//Handle Exceptions
//e.printStackTrace();
}
}
Run your program. Move your hand above the Distance Phidget to modify the frequency of the sound.>
Data Interval
If you haven’t already, make sure to check out the Advanced Lesson on Data Interval to understand the code.
Mapping
In the code above, the map function is used to map the Distance Phidget output to a reasonable frequency range. You can learn more about the map function here.
Practice
- Try modifying the maximum frequency to 10,000Hz instead of 1000Hz.
- Try switching from the SinOsc to one of the other oscillators like SawOsc or TriOsc. For more information, view the Sound Library API.
- Add another Distance Phidget to port 5 on the VINT Hub. Use that sensor to control the volume of the sine object. Hint: modify the amp property.