Temperature Ranking
Paper coffee cups generate a massive amount of trash every year. As more and more people search for an alternative to the throwaway cup, the reusable travel mug selection has increased. Travel mugs can also keep your beverage warm for longer than a paper cup. In this project, you will use a Thermocouple Phidget to determine which travel mug keeps your beverage the warmest.
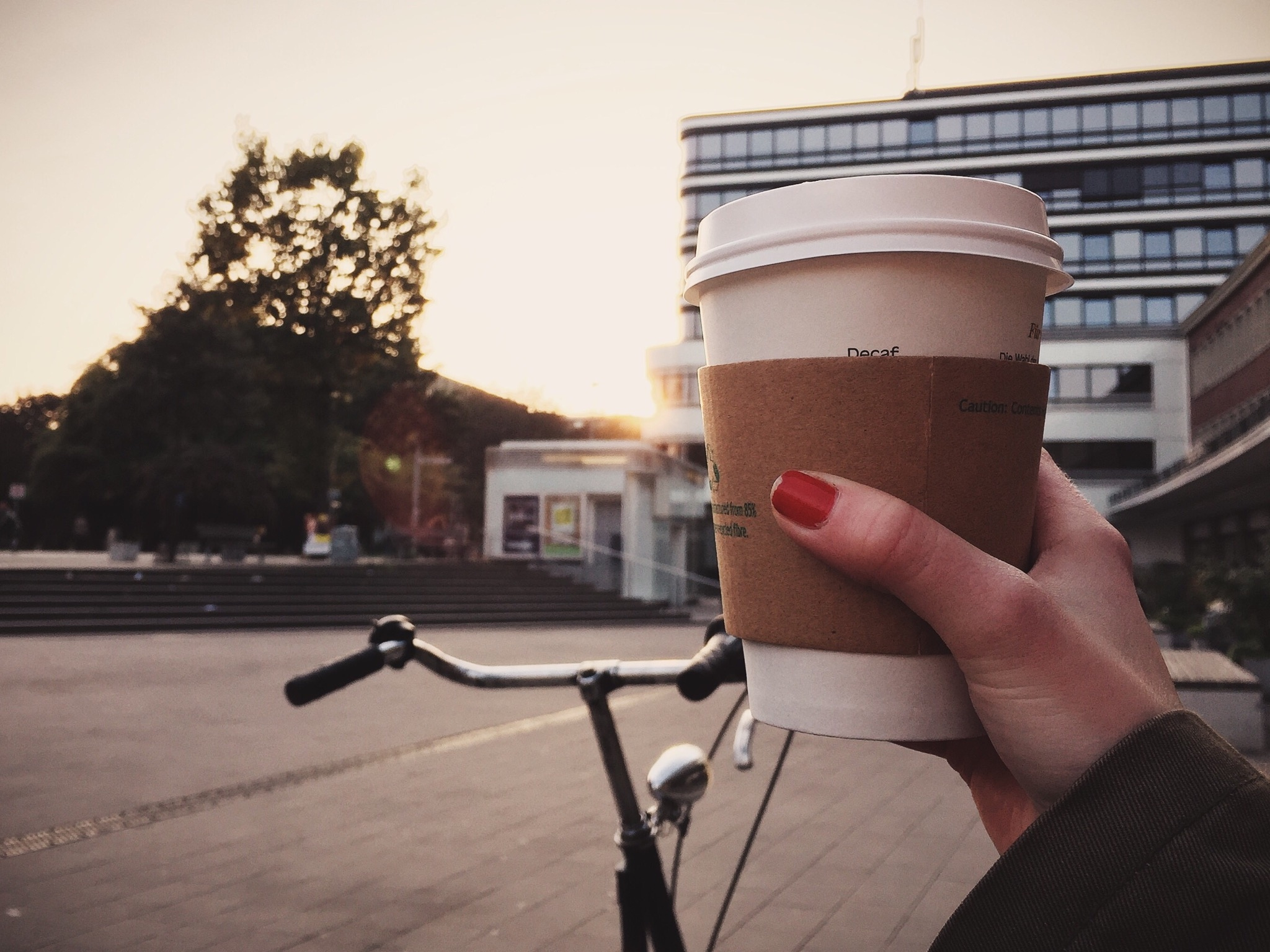
Setup
Before getting started, make sure you have the following parts.
VINT Hub
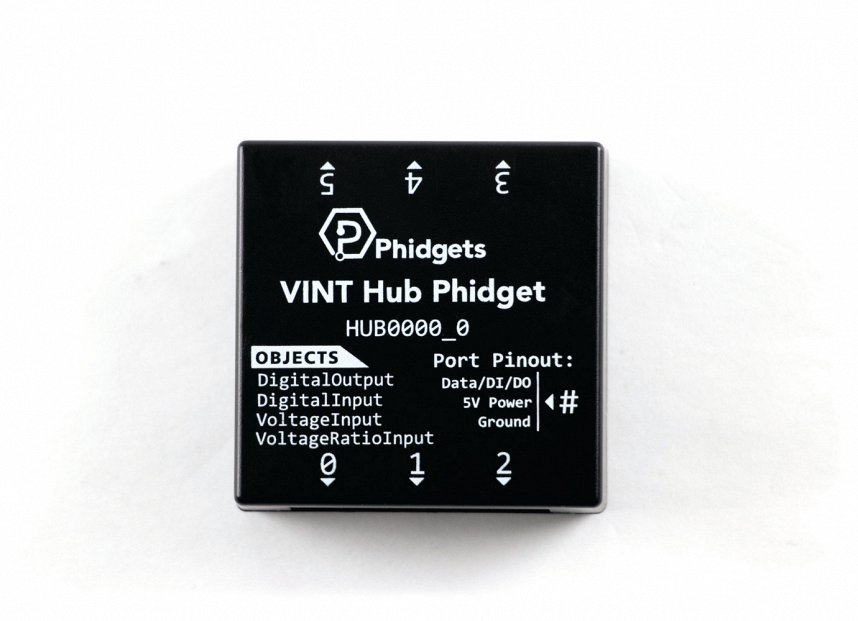
Flathead Screwdriver
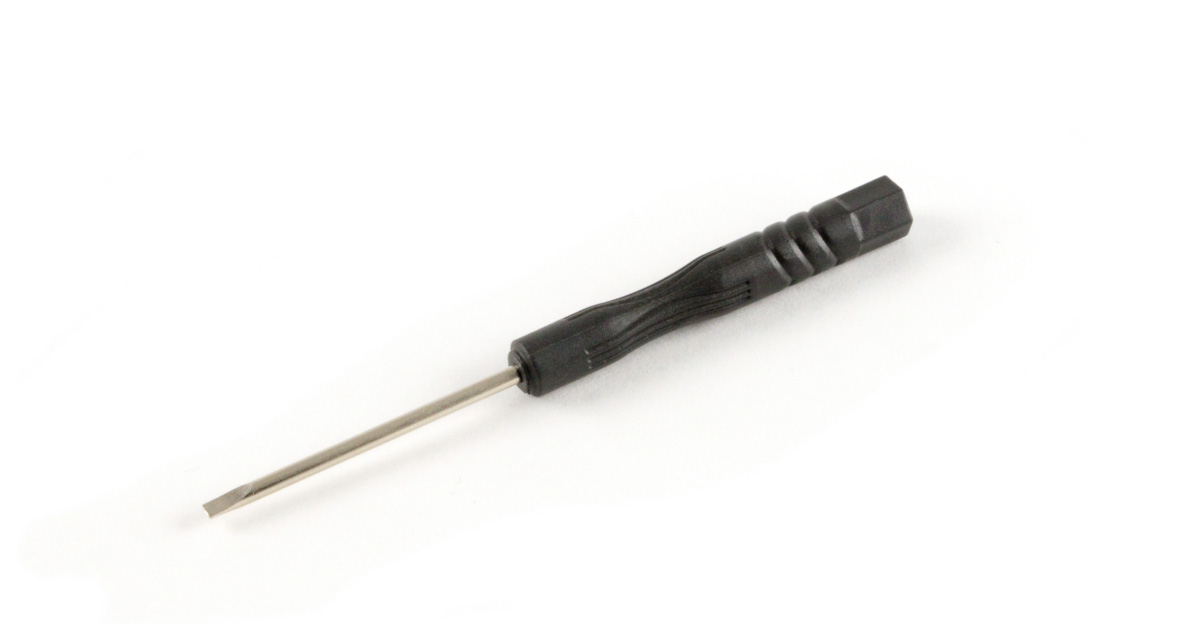
Step 1
Attach Thermocouple Phidget to the VINT Hub and VINT Hub to your computer.
Connect the thermocouple wires to the Phidget with a screwdriver (red = +, white = -).
Step 2
Optional, boil water and pour it into the cups, this will give the liquids time to cool.
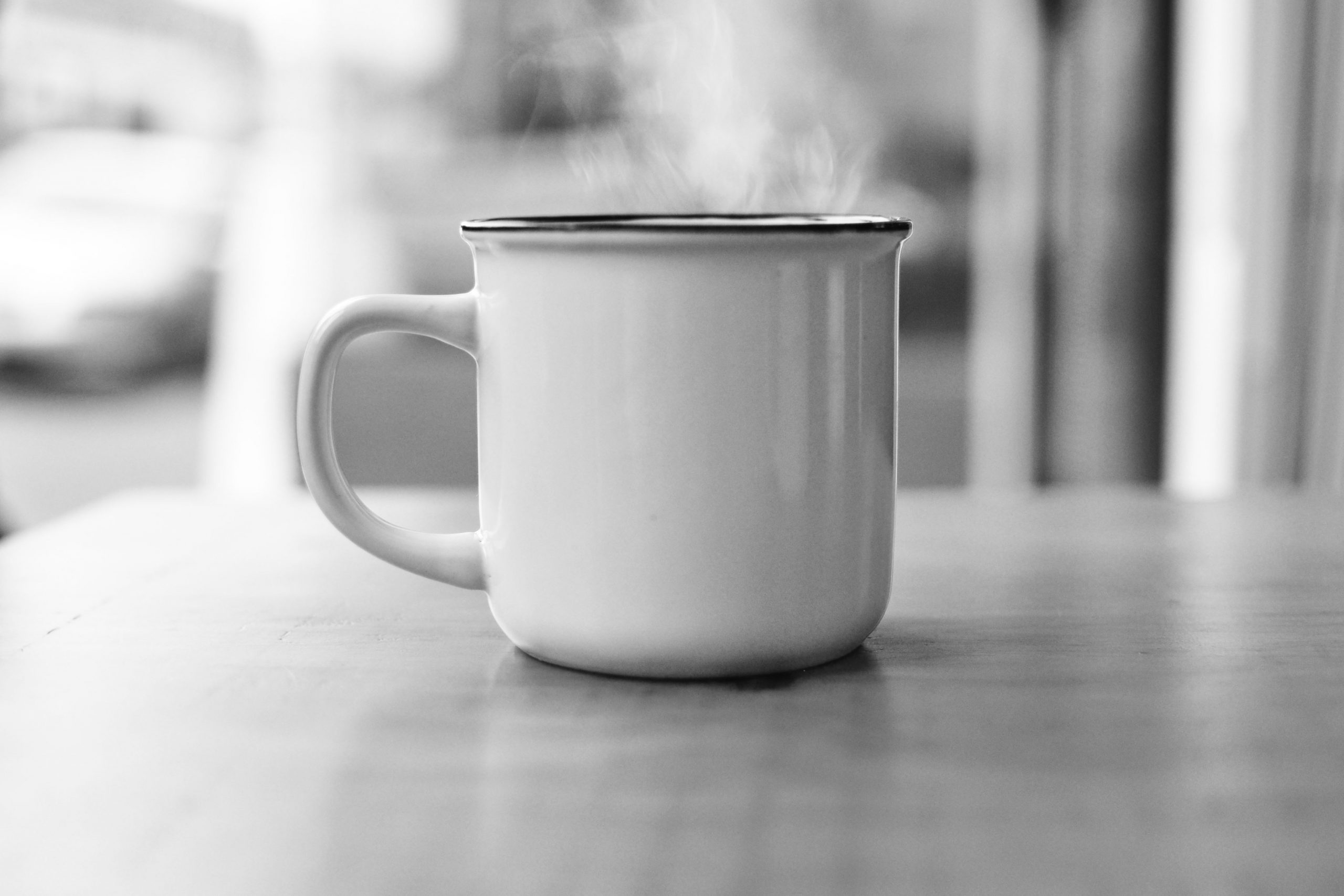
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
package temperatureranking;
//Add Phidgets Library
import com.phidget22.*;
public class TemperatureRanking {
public static void main(String[] args) throws Exception{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Use your Phidget
while(true){
System.in.read();
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C");
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class TemperatureRanking {
public static void main(String[] args) throws Exception{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Use your Phidget
while(true){
System.in.read();
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C");
Thread.sleep(150);
}
}
}
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
#Required for sleep statement
import time
#Create
temperatureSensor = TemperatureSensor()
#Open
temperatureSensor.openWaitForAttachment(1000)
#Use your Phidget
while (True):
input("Press Enter to measure")
print("Temperature: " + str(temperatureSensor.getTemperature()) + " °C")
time.sleep(0.15)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace TemperatureRanking{
class Program{
static void Main(string[] args){
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.Open(1000);
//Use your Phidgets
while(true){
//Press enter to read temperature
Console.ReadKey();
System.Console.WriteLine("Temperature: " + temperatureSensor.Temperature + " °C");
System.Threading.Thread.Sleep(150);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course. Insert the code below.
Not your programming language? Set your preferences so we can display relevant code examples
import Cocoa
//Add Phidget Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let temperatureSensor = TemperatureSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Open
try temperatureSensor.open()
}catch{
print(error)
}
}
@IBAction func getTemperature(_ sender: Any) {
DispatchQueue.main.async {
do{
//Record Temperature
let temperature = try self.temperatureSensor.getTemperature()
self.sensorLabel.stringValue = String(temperature) + " °C"
}catch{
print(error)
}
}
}
}
- Run code. The temperature is recorded when the enter key is pressed.
- Record the temperature of 5 students' hands by having each student hold the end of the thermocouple for a moment then record the temperature.
- Pour hot water into 5 different cups, wait 15 minutes, and then measure the temperatures of each of the liquids using the thermocouple.
- Run code, Temperatures are recorded when the enter key is pressed.
- Record the temperature of 5 students' hands by having each student hold the end of the thermocouple for a moment then record the temperature.
- Pour hot water into 5 different cups, wait 15 minutes, and then measure the temperatures of each of the liquids using the thermocouple.
- Run code, Temperatures are recorded when the enter key is pressed.
- Record the temperature of 5 students' hands by having each student hold the end of the thermocouple for a moment then record the temperature.
- Pour hot water into 5 different cups, wait 15 minutes, and then measure the temperatures of each of the liquids using the thermocouple.
- Run code, Temperatures are recorded when the button is pressed.
- Record the temperature of 5 students' hands by having each student hold the end of the thermocouple for a moment then record the temperature.
- Pour hot water into 5 different cups, wait 15 minutes, and then measure the temperatures of each of the liquids using the thermocouple.
Practice
- Add an array to record 5 different temperatures. Your program should exit after 5 temperatures have been recorded (user pressed enter 5 times).