Schedule Tasks With Cron
(Raspberry Pi)
It can be useful for certain programs to run on a schedule instead of having them constantly run in the background. For example, if you are monitoring the soil moisture of a plant, you may want to take a sample every minute, every 60 minutes, or even just once a day.
This project will show you how to schedule a Python program to run every minute using cron on the Raspberry Pi.
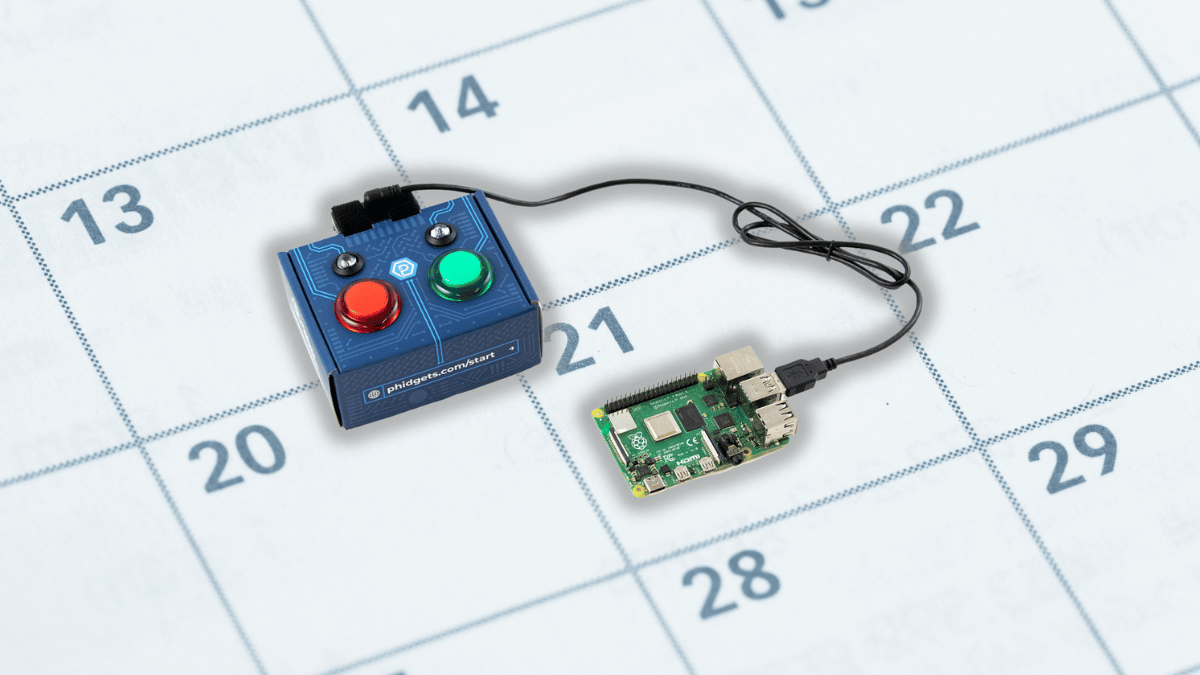
Setup
Before getting started, make sure you have the following parts.
Step 1
Simply connect your Getting Started Kit to your Raspberry Pi.
If you haven't set up your Raspberry Pi with Phidgets yet, visit the Getting Started Kit tutorial.
Write Code
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
#Add Phidgets library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
#Required for sleep statement
import time
#Create
temperatureSensor = TemperatureSensor()
#Open
temperatureSensor.openWaitForAttachment(1000)
#Write data to file in CSV format
with open ('phidgets_temperature.csv','a') as datafile:
datafile.write(str(temperatureSensor.getTemperature()) + "\\n")
Create Program
The code above logs temperature to a file and then exits.
Save the Python script in /home/pi/ under the name phidgets_temperature.py. If you are unfamiliar with logging data from a Phidgets sensor, review this project.
Schedule With Cron
Now that we have a simple program, we will schedule it to run every minute using cron. cron is a scheduling utility that allows you to run tasks (called cron jobs) at a specified frequency.
To create a cron job, open your terminal and enter the following command:
sudo crontab -e
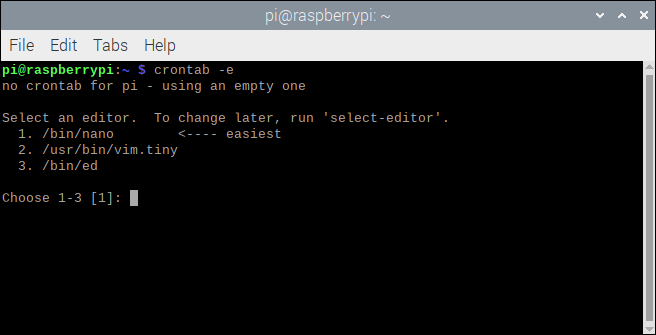
If this is the first time using cron, you may be prompted to select an editor. We recommend selecting the nano editor.
After the cron file is created, scroll down to the bottom of the file and enter the following command:
* * * * * python3 /home/pi/phidgets_temperature.py
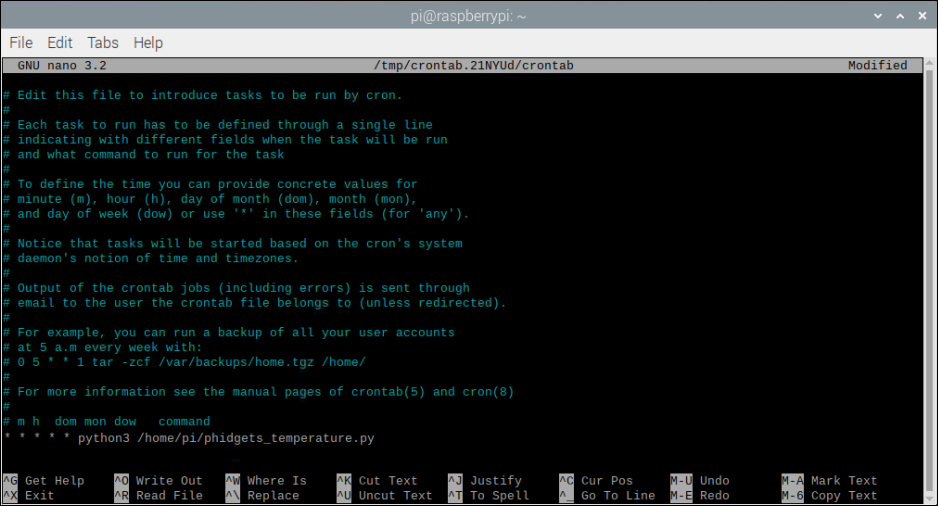
Press Ctrl-X to exit. You will be prompted to save the file, press Y for Yes and then Enter.
Your file is now saved, and your Python program will run every minute! You can easily modify how often your program runs by editing the cron file. Here are a few examples:
*/5 * * * * python3 /home/pi/phidgets_temperature.py #runs every 5 minutes
0 * * * * python3 /home/pi/phidgets_temperature.py #runs once every hour
0 0 * * * python3 /home/pi/phidgets_temperature.py #runs once every day
To experiment with different times, visit crontab.guru.