Start Automatically
(Raspberry Pi)
It can be very useful to have your programs start automatically when your Raspberry Pi launches. For example, you may be logging data from a greenhouse and displaying it on a website. After developing the program, you will disconnect your Raspberry Pi from keyboards/monitors, plug it in and let it log data and serve your website.
This project will show you how to run your projects automatically when your Raspberry Pi starts up using a system service.
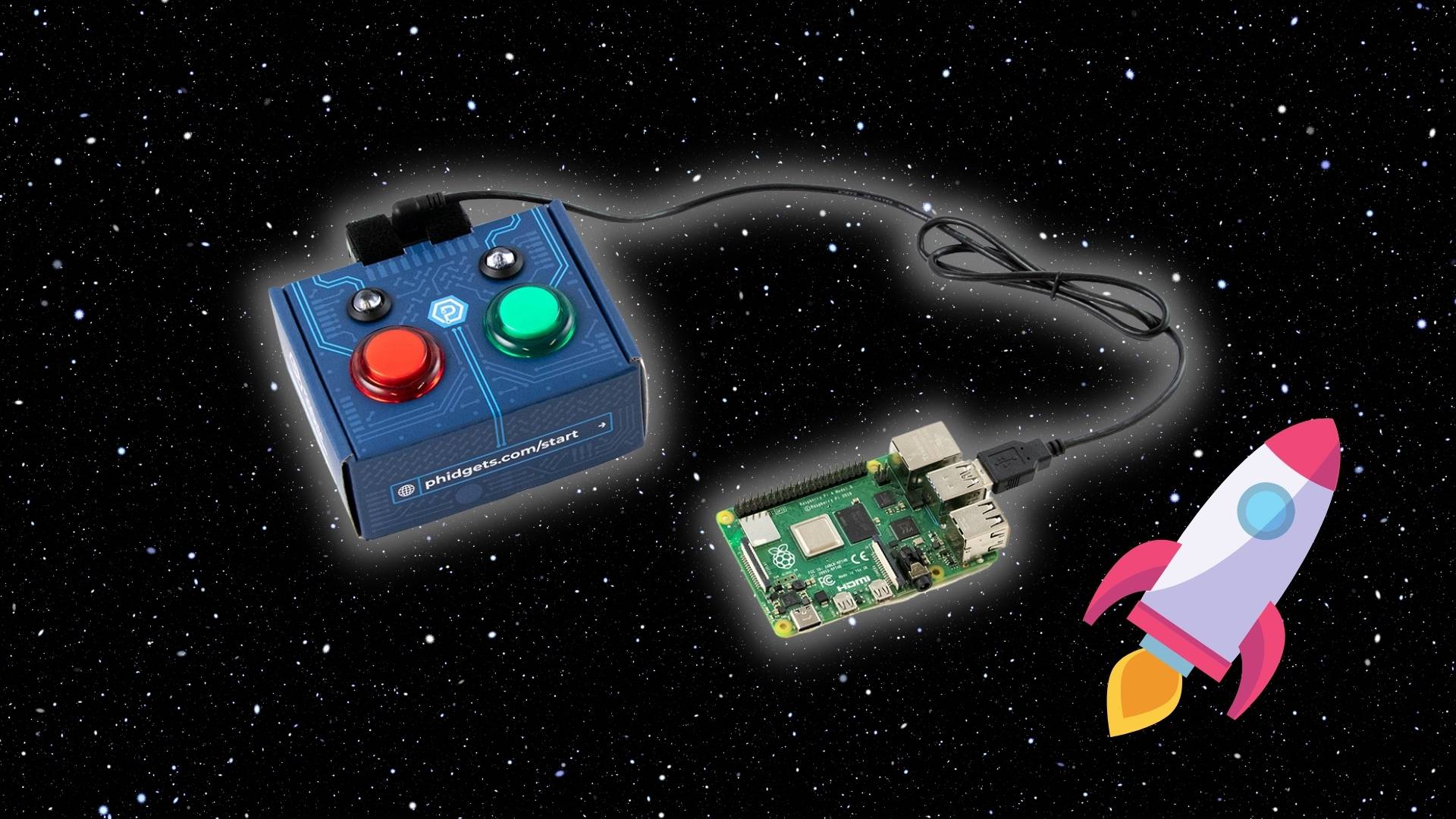
Setup
Before getting started, make sure you have the following parts.
Step 1
Simply connect your Getting Started Kit to your Raspberry Pi.
If you haven't set up your Raspberry Pi with Phidgets yet, visit the Getting Started Kit tutorial.
Write Code
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
#Add Phidgets library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
from Phidget22.Devices.DigitalOutput import *
#Required for sleep statement
import time
#Create
temperatureSensor = TemperatureSensor()
statusLED = DigitalOutput()
#Address
statusLED.setHubPort(1)
statusLED.setIsHubPortDevice(True)
#Open
temperatureSensor.openWaitForAttachment(1000)
statusLED.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
#Update user
print("Logging data...")
#Blink LED to indicate program is running
statusLED.setState(not statusLED.getState())
#Write data to file in CSV format
with open ('phidgets_temperature.csv','a') as datafile:
datafile.write(str(temperatureSensor.getTemperature()) + "\n")
time.sleep(1.0)
Create Program
The code above logs temperature to a file and flashes the red LED on your Getting Started Kit.
Save the Python script in /home/pi/ under the name phidgets_temperature.py. If you are unfamiliar with logging data from a Phidgets sensor, review this project.
Create a System Service
Services allow you to run programs in the background without having to manually start them. In this situation, we would like our program to run automatically when the Raspberry Pi boots, so a service will be a good choice.
Open your terminal and enter the following command:
sudo nano /etc/systemd/system/phidgettemperature.service
This will create a new file at the location /etc/systemd/system/ called phidgettemperature.service. You can also navigate to this location using the graphical user interface and create the file yourself.
Next, paste the code below into your new file and save it.
# Contents of /etc/systemd/system/phidgettemperature.service
[Unit]
Description=Phidget Temperature Logger
After=network.target
[Service]
Type=simple
Restart=always
WorkingDirectory=/home/pi/
User=pi
ExecStart=/usr/bin/python3 /home/pi/Desktop/phidgets_temperature.py
[Install]
WantedBy=multi-user.target
To learn more about what each line of the service accomplishes, visit this page.
To start your service, copy the following commands into your terminal.
systemctl daemon-reload
systemctl enable phidgettemperature.service
systemctl start phidgettemperature.service
The service is now enabled, and will start every time the Raspberry Pi boots. You can view the status of your service by entering the following command.
systemctl status phidgettemperature.service