Prerequisites
This project assumes you are familiar with the following
Setup
Make sure you have all the parts before moving on.
VINT Hub
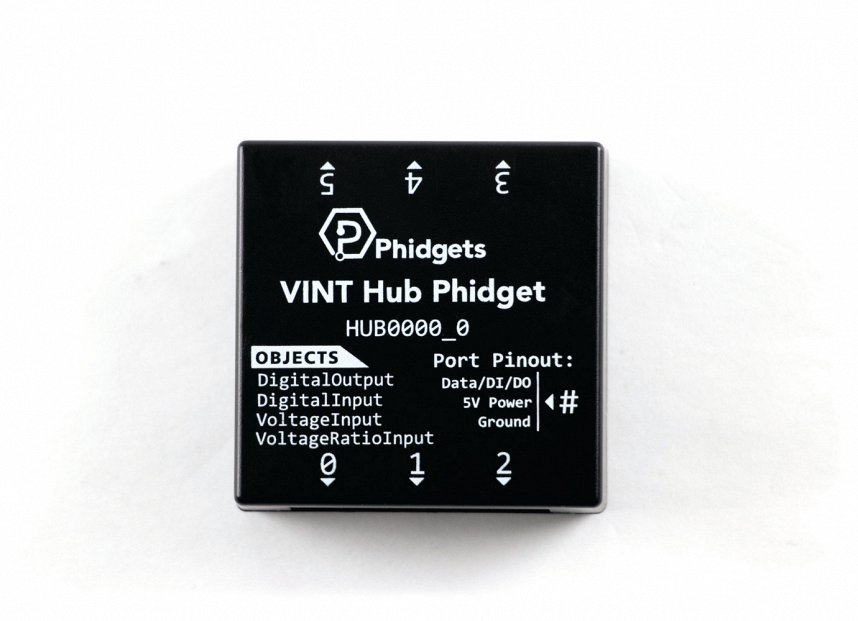
Install pynput
In order to use pynput, you first have to install it. You do this in the same way you previously installed the Phidget22 library. Simply navigate to your package manager, search for pynput and press install!
PyCharm
If you're using PyCharm, select File > Settings > Python Interpreter and use the + symbol to install pynput.
PyScripter
If you're using PyScripter, select Tools > Tools > Install Packages with pip and enter pynput.
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
#Add Phidgets Library
from Phidget22.Devices import *
from Phidget22.Devices.SoundSensor import *
from pynput.mouse import Button, Controller
#Required for sleep statement
import time
#Sound Sensor Event
def onSPLChange(self, dB, dBA, dBC, Octaves):
if (dB > 70): #modify this value based on how loud the sound is
mouse.press(Button.left)
else:
mouse.release(Button.left)
#Create
mouse = Controller() #this is from the pynput library
soundSensor = SoundSensor()
#Subscribe to Events
soundSensor.setOnSPLChangeHandler(onSPLChange)
#Open
soundSensor.openWaitForAttachment(1000)
#Set Data Interval to minimum | This will increase the data rate from the sensor to make your program more responsive
soundSensor.setDataInterval(soundSensor.getMinDataInterval())
while True:
# loop forever
time.sleep(0.1)
Run Your Program
Find a game similar to Flappy Bird that uses the mouse as input:
- Example: Helicopter Game.
Run your Python program and move your mouse pointer over the play area. Making a loud noise will start the game. From there continuing to make noise will move the helicopter up, and stopping will cause it to fall.
Practice
- Try using specific frequencies from the Sound Phidget to move the helicopter.