Mood Sensing
A mood ring is a popular trinket that claims to “sense” your mood when you place it on your finger. In reality, the ring contains a thermochromic substance that changes color based on temperature. In this project, you will create your own mood sensing device using a Humidity Phidget and Processing!
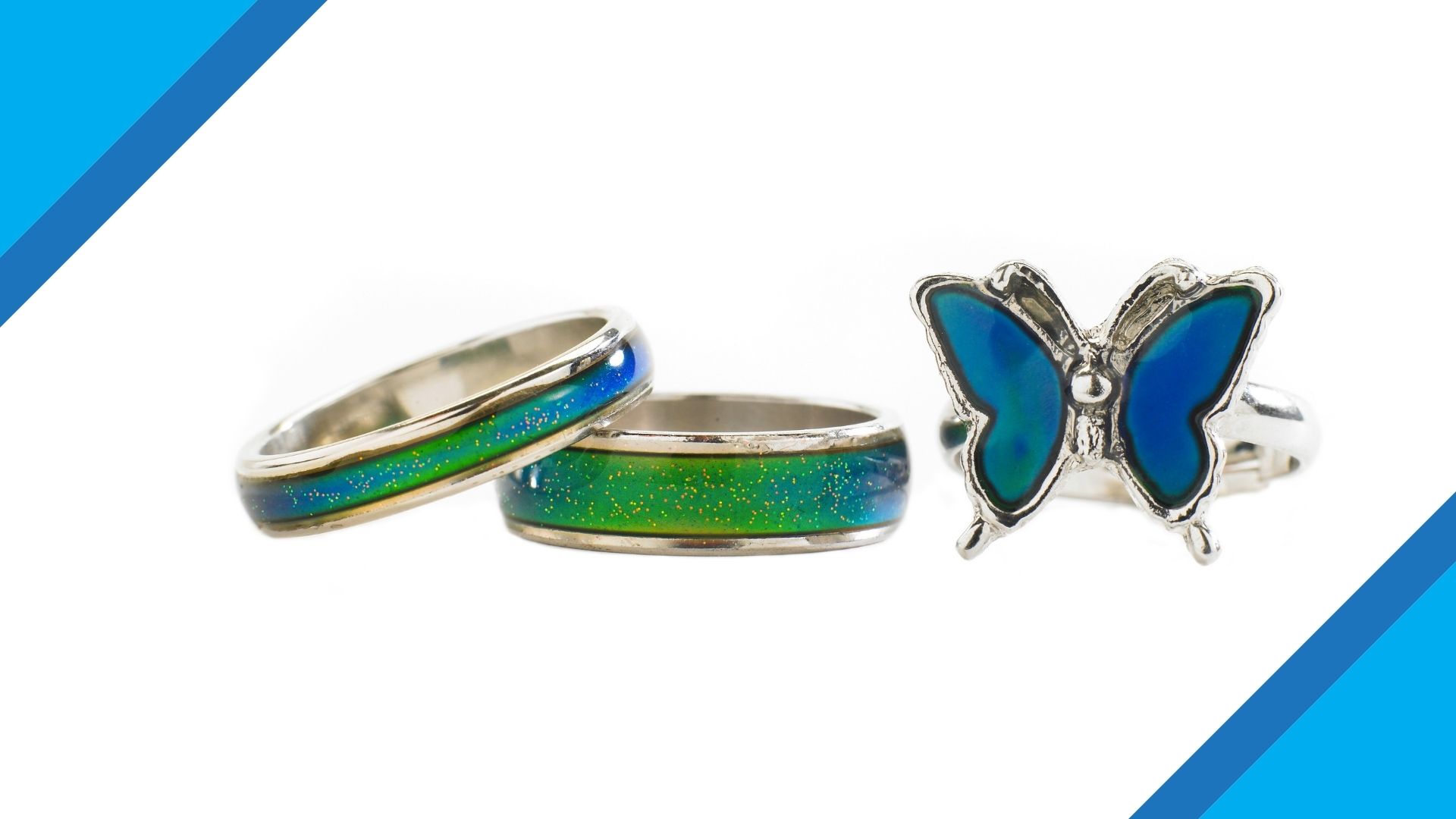
Setup
Before getting started, make sure you have the following parts.
VINT Hub
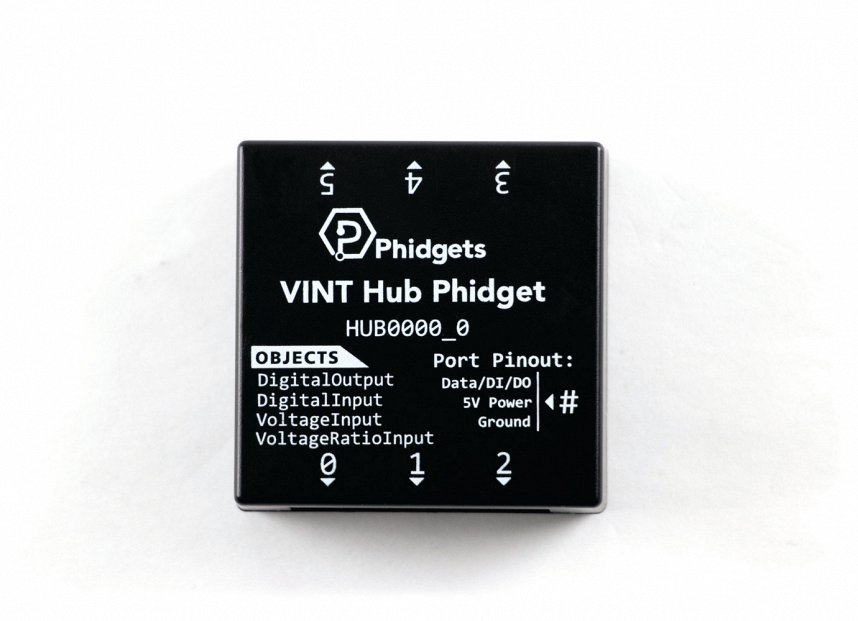
Write Code
Copy the code below into a new Processing project. If you need a reminder of how to do this, revisit the Getting Started Course.
//Add Phidgets Library
import com.phidget22.*;
//Define
TemperatureSensor temperature;
void setup(){
size(400,400);
try{
//Create
temperature = new TemperatureSensor();
//Open
temperature.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
double mood = temperature.getTemperature();
//Convert mood to Farenheit
//mood = (mood * 1.8) + 32;
//if cold, turn blue, else, turn red
if(mood < 23){
background(#0000ff);
}
else{
background(#ff0000);
}
delay(150);
}catch(Exception e){
e.printStackTrace();
}
}
Run your program. The background will display a blue color or a red color depending on the temperature. You can hold the Humidity Phidget tightly in your hand to increase the temperature.
Practice
- Modify your code to include the following colors. Blue should be neutral, so depending on your room, you may have to modify the chart.
Temperature (C) | Temperature (F) | Color | Hex Value |
---|---|---|---|
20 | 68 | Black | #000000 |
21 | 69.8 | Orange | ? |
22 | 71.6 | Yellow | ? |
23 | 73.4 | Blue (Neutral) | #0000ff |
24 | 75.2 | Green | ? |
2. Add text to the screen to express the current mood. The mood should range from “Stressed Out” for Black to “Blissful” for Red with Blue reading “Relaxed”.