Setup
Connect your Spatial Phidget to your computer.
VINT Hub
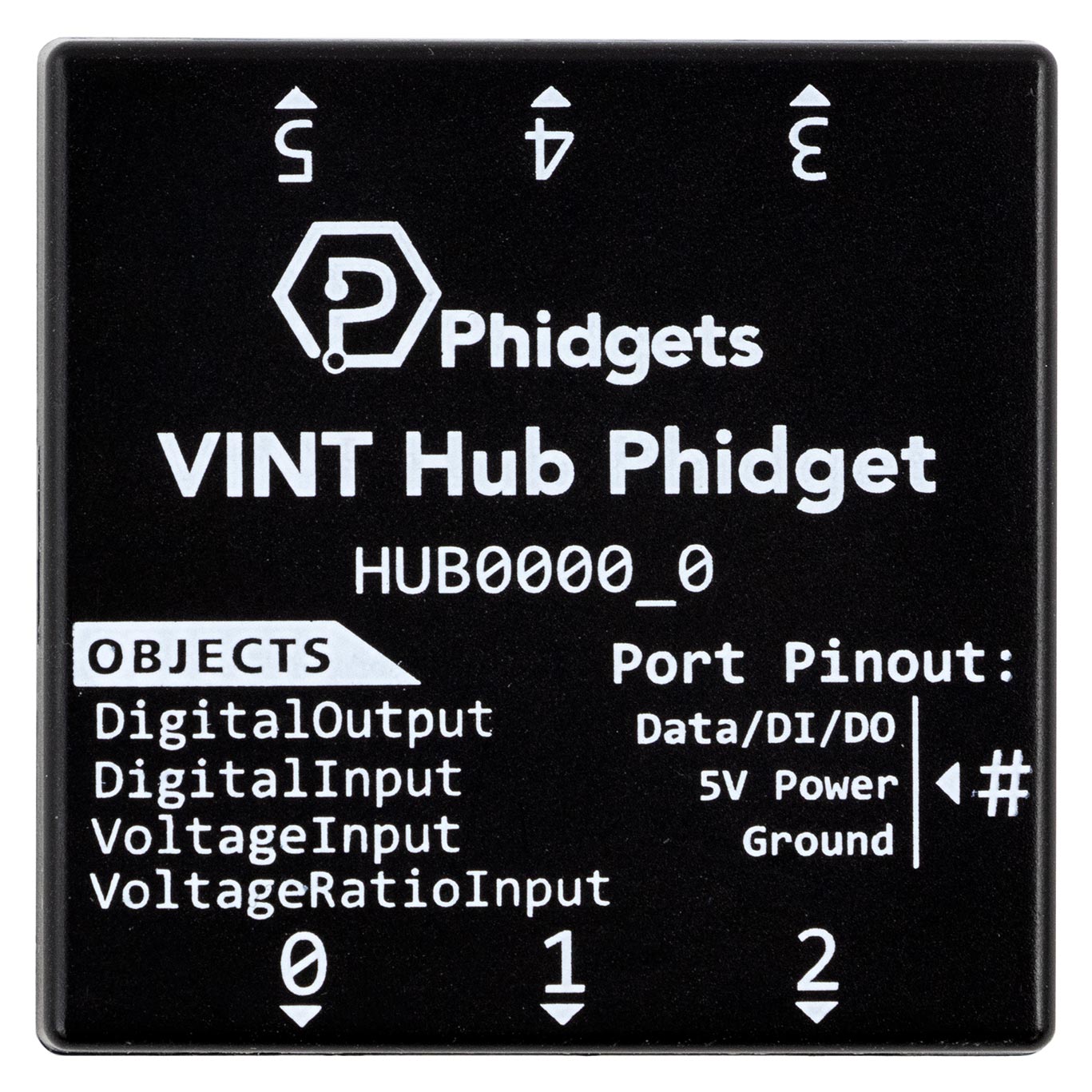
Write code (Java)
Copy the code below into a new Java project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
import com.phidget22.*;
public class CompassHeading {
public static void main(String[] args) throws Exception {
//Create
Spatial spatial = new Spatial();
//Open
spatial.open(1000);
//Use your Phidgets
while (true) {
try{
double compassHeading = spatial.getEulerAngles().heading;
System.out.println("Compass Heading: " + Math.round(compassHeading) + "°");
if (compassHeading >= -5 && compassHeading <= 5) {
System.out.println("\nPOINTING NORTH\n");
} else if (compassHeading >= 85 && compassHeading <= 95) {
System.out.println("\nPOINTING EAST\n");
} else if (compassHeading <= -175 || compassHeading >= 175) {
System.out.println("\nPOINTING SOUTH\n");
} else if (compassHeading <= -85 && compassHeading >= -95) {
System.out.println("\nPOINTING WEST\n");
}
}catch(Exception e){
System.out.println("Compass heading not yet calculated.");
}
Thread.sleep(200);
}
}
}
package compassheading;
//Add Phidgets Library
import com.phidget22.*;
public class CompassHeading {
public static void main(String[] args) throws Exception {
//Create
Spatial spatial = new Spatial();
//Open
spatial.open(1000);
//Use your Phidgets
while (true) {
try{
double compassHeading = spatial.getEulerAngles().heading;
System.out.println("Compass Heading: " + Math.round(compassHeading) + "°");
if (compassHeading >= -5 && compassHeading <= 5) {
System.out.println("\nPOINTING NORTH\n");
} else if (compassHeading >= 85 && compassHeading <= 95) {
System.out.println("\nPOINTING EAST\n");
} else if (compassHeading <= -175 || compassHeading >= 175) {
System.out.println("\nPOINTING SOUTH\n");
} else if (compassHeading <= -85 && compassHeading >= -95) {
System.out.println("\nPOINTING WEST\n");
}
}catch(Exception e){
System.out.println("Compass heading not yet calculated.");
}
Thread.sleep(200);
}
}
}
Code is not available for this project.
Write code (Python)
Copy the code below into a new Python project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.Spatial import *
#Required for sleep statement
import time
#Create
spatial = Spatial()
#Open
spatial.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
try:
compassHeading = spatial.getEulerAngles().heading
print("Compass Heading: " + str(round(compassHeading)) + "°")
if(compassHeading >= -5 and compassHeading <= 5):
print("\nPOINTING NORTH\n")
elif(compassHeading >= 85 and compassHeading <= 95):
print("\nPOINTING EAST\n")
elif(compassHeading <= -175 or compassHeading >= 175):
print("\nPOINTING SOUTH\n")
elif(compassHeading <= -85 and compassHeading >= -95):
print("\nPOINTING WEST\n")
except:
print("Compass heading not yet calculated.")
time.sleep(0.2)
Write code (C#)
Copy the code below into a new C# project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
using Phidget22;
namespace CompassHeading
{
internal class Program
{
static void Main(string[] args)
{
//Create
Spatial spatial = new Spatial();
//Open
spatial.Open(1000);
//Use your Phidgets
while (true)
{
try
{
double compassHeading = spatial.EulerAngles.Heading;
Console.WriteLine("Compass Heading: " + Math.Round(compassHeading) + "°");
if (compassHeading >= -5 && compassHeading <= 5)
{
Console.WriteLine("\nPOINTING NORTH\n");
}
else if (compassHeading >= 85 && compassHeading <= 95)
{
Console.WriteLine("\nPOINTING EAST\n");
}
else if (compassHeading <= -175 || compassHeading >= 175)
{
Console.WriteLine("\nPOINTING SOUTH\n");
}
else if (compassHeading <= -85 && compassHeading >= -95)
{
Console.WriteLine("\nPOINTING WEST\n");
}
}
catch (Exception ex)
{
Console.WriteLine("Compass heading not yet calculated");
}
System.Threading.Thread.Sleep(200);
}
}
}
}
Write code (Swift)
Copy the code below into a new Swift project. If you need a reminder of how to do this, revisit the Getting Started Course.
Not your programming language? Set your preferences so we can display relevant code examples
Code not available.
Run Your Program
Slowly spin your Spatial Phidget on your desk. You will see a Compass Heading being printed to the screen. This value ranges from 0 to ±180 degrees. When you are within 10 degrees of a cardinal direction (N,E,S,W) your program will print the direction it is pointing.
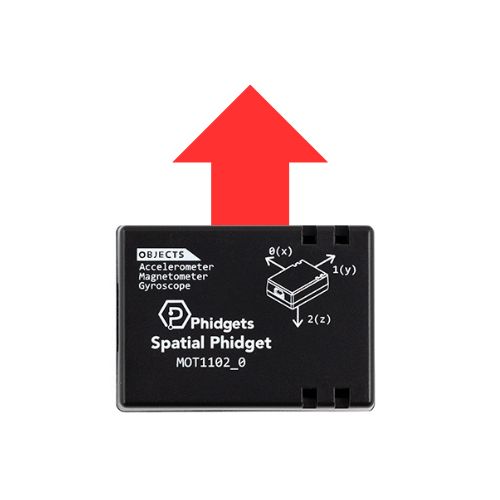
The Compass Heading is aligned with the top of your Spatial Phidget (shown above).
Practice
- Modify your code to print the ordinal directions (NE, NW, SE, SW)
- Create a GUI and point an arrow in the direction your Spatial Phidget is pointing.