Smart Phidget Events
In this lesson, you’ll learn how to write a program that gets both temperature and humidity data using events!
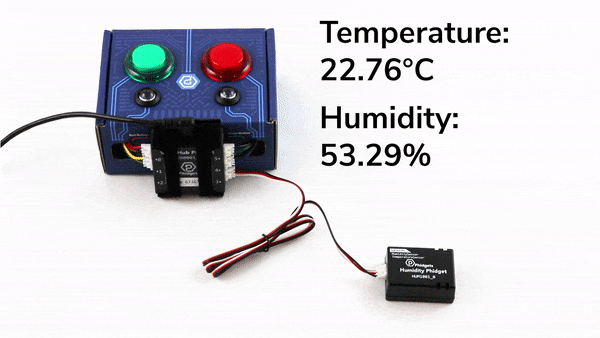
Write Code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception {
//Create
HumiditySensor humiditySensor = new HumiditySensor();
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Humidity Event | Event code runs when data input from the sensor changes. The following event is a Humidity change event. The contained code will only run when the humidity input changes.
humiditySensor.addHumidityChangeListener(new HumiditySensorHumidityChangeListener() {
public void onHumidityChange(HumiditySensorHumidityChangeEvent e) {
System.out.println("Humidity: " + e.getHumidity() + "%RH");
}
});
//Temperature Event | Event code runs when data input from the sensor changes. The following event is a Temperature change event. The contained code will only run when the temperature input changes.
temperatureSensor.addTemperatureChangeListener(new TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
System.out.println("Temperature: " + e.getTemperature() + "°C");
}
});
//Open
humiditySensor.open(1000);
temperatureSensor.open(1000);
//Keep program running
while (true) {
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception {
//Create
HumiditySensor humiditySensor = new HumiditySensor();
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Humidity Event | Event code runs when data input from the sensor changes. The following event is a Humidity change event. The contained code will only run when the humidity input changes.
humiditySensor.addHumidityChangeListener(new HumiditySensorHumidityChangeListener() {
public void onHumidityChange(HumiditySensorHumidityChangeEvent e) {
System.out.println("Humidity: " + e.getHumidity() + "%RH");
}
});
//Temperature Event | Event code runs when data input from the sensor changes. The following event is a Temperature change event. The contained code will only run when the temperature input changes.
temperatureSensor.addTemperatureChangeListener(new TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
System.out.println("Temperature: " + e.getTemperature() + "°C");
}
});
//Open
humiditySensor.open(1000);
temperatureSensor.open(1000);
//Keep program running
while (true) {
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
TemperatureSensor temperatureSensor;
HumiditySensor humiditySensor;
void setup(){
try{
//Create
humiditySensor = new HumiditySensor();
temperatureSensor = new TemperatureSensor();
//Humidity Event | Event code runs when data input from the sensor changes. The following event is a Humidity change event. The contained code will only run when the humidity input changes.
humiditySensor.addHumidityChangeListener(new HumiditySensorHumidityChangeListener() {
public void onHumidityChange(HumiditySensorHumidityChangeEvent e) {
System.out.println("Humidity: " + e.getHumidity() + "%RH");
}
});
//Temperature Event | Event code runs when data input from the sensor changes. The following event is a Temperature change event. The contained code will only run when the temperature input changes.
temperatureSensor.addTemperatureChangeListener(new TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
System.out.println("Temperature: " + e.getTemperature() + "°C");
}
});
//Open
humiditySensor.open(1000);
temperatureSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
delay(150);
}
Write Code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.HumiditySensor import *
from Phidget22.Devices.TemperatureSensor import *
import time
#Humidity Change Event | Event code runs when data input from the sensor changes. The following event is a Humidity change event. The contained code will only run when the humidity input changes.
def onHumiditySensor_HumidityChange(self, humidity):
print("Humidity: " + str(humidity) + "%RH")
#Temperature Change Event | Event code runs when data input from the sensor changes. The following event is a Temperature change event. The contained code will only run when the temperature input changes.
def onTemperatureSensor_TemperatureChange(self, temperature):
print("Temperature: " + str(temperature) + "°C")
#Create
humiditySensor = HumiditySensor()
temperatureSensor = TemperatureSensor()
#Subscribe to event | Subscribing to an Event tells your program to start listening to a Phidget. Here, the program will begin to listen to the temperature and humidity sensors in you Humidity Phidget
humiditySensor.setOnHumidityChangeHandler(onHumiditySensor_HumidityChange)
temperatureSensor.setOnTemperatureChangeHandler(onTemperatureSensor_TemperatureChange)
#Open
humiditySensor.openWaitForAttachment(1000)
temperatureSensor.openWaitForAttachment(1000)
# Keep program running
while(True):
time.sleep(0.15)
Write Code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
using Phidget22.Events;
namespace GettingStarted
{
class Program
{
//Humidity Change Event | Event code runs when data input from the sensor changes. The following event is a Humidity change event. The contained code will only run when the humidity input changes.
private static void HumiditySensor_HumidityChange(object sender, Phidget22.Events.HumiditySensorHumidityChangeEventArgs e)
{
System.Console.WriteLine("Humidity: " + e.Humidity + "%RH");
}
//Temperature Change Event | Event code runs when data input from the sensor changes. The following event is a Temperature change event. The contained code will only run when the temperature input changes.
private static void TemperatureSensor_TemperatureChange(object sender, Phidget22.Events.TemperatureSensorTemperatureChangeEventArgs e)
{
System.Console.WriteLine("Temperature: " + e.Temperature + "°C");
}
static void Main(string[] args)
{
//Create
HumiditySensor humiditySensor = new HumiditySensor();
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Subscribe to event | Subscribing to an Event tells your program to start listening to a Phidget. Here, the program will begin to listen to the temperature and humidity sensors in you Humidity Phidget
humiditySensor.HumidityChange += HumiditySensor_HumidityChange;
temperatureSensor.TemperatureChange += TemperatureSensor_TemperatureChange;
//Open
humiditySensor.Open(1000);
temperatureSensor.Open(1000);
//Keep Program Running
while (true)
{
System.Threading.Thread.Sleep(150);
}
}
}
}
Write Code (Swift)
Oops, how did you end up here? There is no Button Events lesson for Swift. Go back to the main tutorial page.
Run Your Program
You will see the temperature and humidity displayed.
What are Events?
In previous programs, you used a method called polling to access data from your sensor. This meant your program was continuously checking the state of the sensor (using a loop) to get the latest value. Events allow you to sit back and wait for data to come to you! For example, in the program above, you simply subscribe to the Temperature Change Event and wait for your function to be called when there is new temperature data available.
Practice
- Compare the code sample to the code sample in Lesson 5. Note the differences in the code and the program’s output.
- Only print the temperature if it is above 21°C (70°F). If the temperature is below 21°C, print "Room is too cold".
Troubleshoot
I am getting a "Timed Out" exception.
- Make sure the USB cable from your VINT Hub to your computer is attached properly.
- Make the Phidget cable (the black, red and white one) is connected to your VINT Hub and to your Humidity Phidget properly).
- Make sure no other program is running that uses Phidgets. If a Phidget is already in use in another program, it will be busy and won't respond to this one.
Still having issues?
Visit the Advanced Troubleshooting Page or contact us (education@phidgets.com).