Attach/Detach Events
In this lesson, you will learn how to use attach and detach events so you can keep track of the Phidgets in your programs!
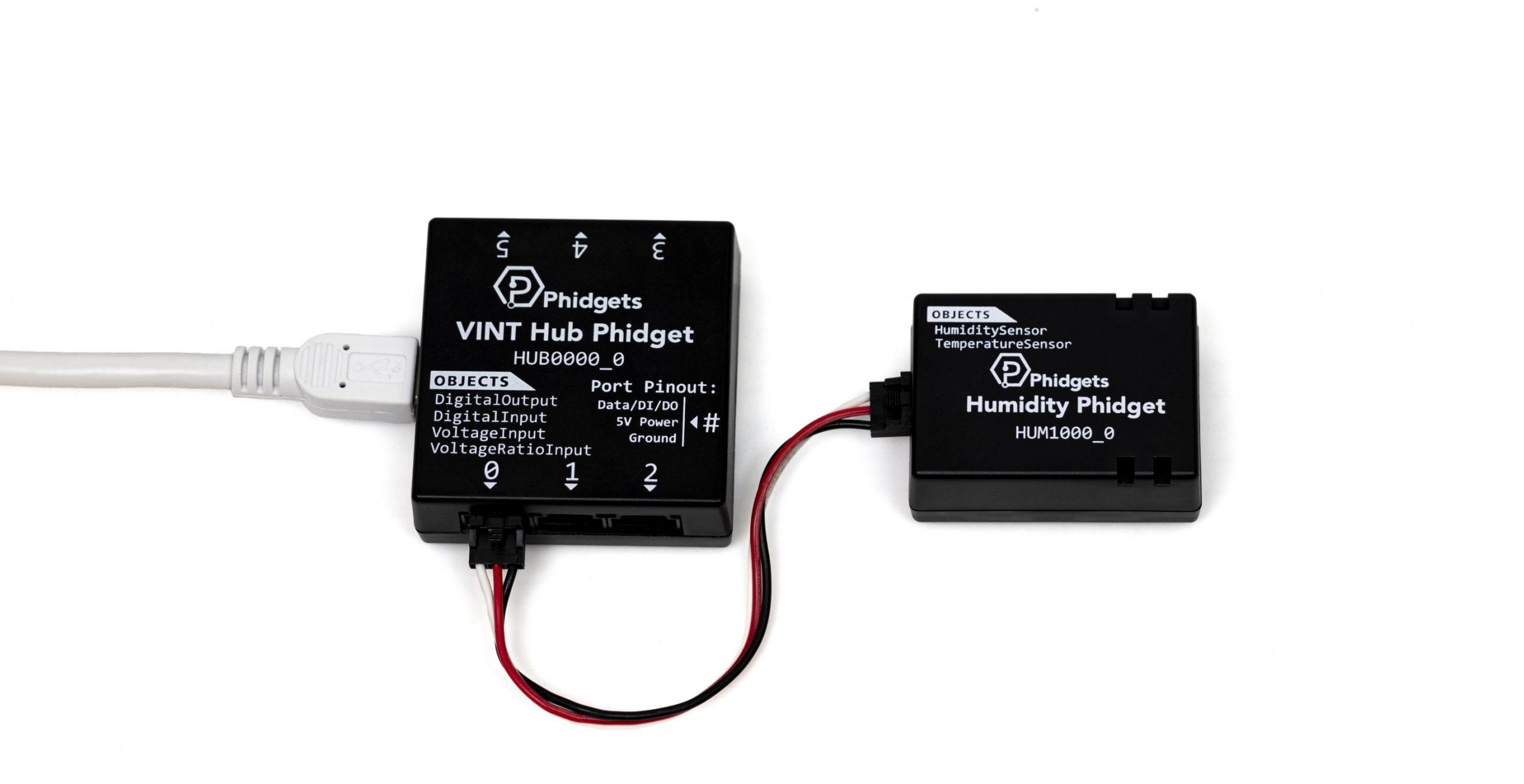
Write Code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception {
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Data Event | Event code runs when data from sensor changes.
temperatureSensor.addTemperatureChangeListener(new TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
//Print temperature
System.out.println("Temperature: " + e.getTemperature() + "°C");
}
});
//Attach Event | Attach Events run when a Phidget is connected to the Object
temperatureSensor.addAttachListener(new AttachListener() {
public void onAttach(AttachEvent e) {
System.out.println("Attach!");
}
});
//Detach Event | Detach Events run when a Phidget is disconnected from the Object
temperatureSensor.addDetachListener(new DetachListener() {
public void onDetach(DetachEvent e) {
System.out.println("Detach!");
}
});
//Open
temperatureSensor.open(1000);
//Keep program running
while (true) {
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception {
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Data Event | Event code runs when data from sensor changes.
temperatureSensor.addTemperatureChangeListener(new TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
//Print temperature
System.out.println("Temperature: " + e.getTemperature() + "°C");
}
});
//Attach Event | Attach Events run when a Phidget is connected to the Object
temperatureSensor.addAttachListener(new AttachListener() {
public void onAttach(AttachEvent e) {
System.out.println("Attach!");
}
});
//Detach Event | Detach Events run when a Phidget is disconnected from the Object
temperatureSensor.addDetachListener(new DetachListener() {
public void onDetach(DetachEvent e) {
System.out.println("Detach!");
}
});
//Open
temperatureSensor.open(1000);
//Keep program running
while (true) {
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
TemperatureSensor temperatureSensor;
void setup(){
try{
//Create
temperatureSensor = new TemperatureSensor();
//Data Event | Event code runs when data from sensor changes.
temperatureSensor.addTemperatureChangeListener(new TemperatureSensorTemperatureChangeListener() {
public void onTemperatureChange(TemperatureSensorTemperatureChangeEvent e) {
System.out.println("Temperature: " + e.getTemperature() + "°C");
}
});
//Attach Event | Attach Events run when a Phidget is connected to the Object
temperatureSensor.addAttachListener(new AttachListener() {
public void onAttach(AttachEvent e) {
System.out.println("Attached!");
}
});
//Detach Event | Detach Events run when a Phidget is disconnected from the Object
temperatureSensor.addDetachListener(new DetachListener() {
public void onDetach(DetachEvent e) {
System.out.println("Detached!");
}
});
//Open
temperatureSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
delay(150);
}
Write Code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
import time
#Data Event | Event code runs when data from sensor changes.
def onTemperatureSensor0_TemperatureChange(self, temperature):
print("Temperature: " + str(temperature) + "°C")
#Attach Event | Attach Events run when a Phidget is connected to the Object
def onTemperatureSensor0_Attach(self):
print("Attach!")
#Detach Event | Detach Events run when a Phidget is disconnected from the Object
def onTemperatureSensor0_Detach(self):
print("Detach!")
#Create
temperatureSensor = TemperatureSensor()
#Subscribe to Data Event
temperatureSensor.setOnTemperatureChangeHandler(onTemperatureSensor0_TemperatureChange)
#Subscribe to Attach Event | Subscribe to Events tell your program to start listening to a Phidget. In this case, the program will begin checking to see if your Object and physical Phidget are connected
temperatureSensor.setOnAttachHandler(onTemperatureSensor0_Attach)
#Subscribe to Detach Event | Subscribe to Events tell your program to start listening to a Phidget. In this case, the program will begin checking to see if your Object and physical Phidget are disconnected
temperatureSensor.setOnDetachHandler(onTemperatureSensor0_Detach)
#Open
temperatureSensor.openWaitForAttachment(1000)
#Keep program running
while(True):
time.sleep(0.15)
Write Code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
using Phidget22.Events;
namespace GettingStarted{
class Program{
//Data Event | Event code runs when data from sensor changes.
private static void TemperatureSensor_TemperatureChange(object sender, Phidget22.Events.TemperatureSensorTemperatureChangeEventArgs e)
{
//Print temperature
System.Console.WriteLine("Temperature: " + e.Temperature + "°C");
}
//Attach Event | Attach Events run when a Phidget is connected to the Object
private static void TemperatureSensor_Attach(object sender, Phidget22.Events.AttachEventArgs e)
{
System.Console.WriteLine("Attach!");
}
//Detach Event | Detach Events run when a Phidget is disconnected from the Object
private static void TemperatureSensor_Detach(object sender, Phidget22.Events.DetachEventArgs e)
{
System.Console.WriteLine("Detach!");
}
static void Main(string[] args)
{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Subscribe to Data Event
temperatureSensor.TemperatureChange += TemperatureSensor_TemperatureChange;
//Subscribe to Attach Event | Subscribe to Events tell your program to start listening to a Phidget. In this case, the program will begin checking to see if your Object and physical Phidget are connected
temperatureSensor.Attach += TemperatureSensor_Attach;
//Subscribe to Detach Event | Subscribe to Events tell your program to start listening to a Phidget. In this case, the program will begin checking to see if your Object and physical Phidget are disconnected
temperatureSensor.Detach += TemperatureSensor_Detach;
//Open
temperatureSensor.Open(1000);
//Keep Program Running
while (true)
{
System.Threading.Thread.Sleep(150);
}
}
}
}
Write Code (Swift)
Create two labels in your window and copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
Create two labels
import Cocoa
//Add Phidgets Library | You used cocoapods to install the Phidget library. The statement below give your program access to that code.
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var attachedLabel: NSTextField!
@IBOutlet weak var temperatureLabel: NSTextField!
//Create | Here you have created a TemperatureSensor object. TemperatureSensor is a class in your Phidgets library that gathers temperature data from your Phidget.
let temperatureSensor = TemperatureSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to events
let _ = temperatureSensor.attach.addHandler(onAttach)
let _ = temperatureSensor.detach.addHandler(onDetach)
let _ = temperatureSensor.temperatureChange.addHandler(onTemperatureChange)
//Open | Open establishes a connection between your object and your physical Phidget.
try temperatureSensor.open()
}catch{
print(error)
}
}
//Data event
func onTemperatureChange(sender:TemperatureSensor, temperature: Double){
DispatchQueue.main.async {
//Use your Phidget | Update the label with the latest temperature data.
self.temperatureLabel.stringValue = String(temperature) + "°C"
}
}
//Attach event
func onAttach(sender:Phidget){
DispatchQueue.main.async {
//Update the label with the attached status.
self.attachedLabel.stringValue = "Attached!"
}
}
//Detach event
func onDetach(sender:Phidget){
DispatchQueue.main.async {
//Update the label with the attached status
self.attachedLabel.stringValue = "Detached!"
}
}
}
Run Your Program
You will immediately see an attach event fire, and then you will see the temperature displayed in degrees Celsius.
When should I use Attach/Detach events?
These events are useful if you need to attach and detach Phidgets while your program is running, disable or enable program features, or monitor the connection to your Phidgets. For example, if you made a video game that used Phidgets as input (something like this), you would want to know if a device got disconnected so you could pause your game!
Practice
- Run the code sample. Disconnect and reconnect your USB cable while the code is running. Note what happens.
- All Phidgets have Attach and Detach Events. Modify your code to add an LED and Button. Add an Attach and Detach Events for the LED and button. In each Attach Event, print “Attach” plus the component that is attached. For each Detach Event, print “Detach” plus the component.
Troubleshoot
I am getting a "Timed Out" exception.
- Make sure the USB cable from your VINT Hub to your computer is attached properly.
- Make the Phidget cable (the black, red and white one) is connected to your VINT Hub and to your Humidity Phidget properly).
- Make sure no other program is running that uses Phidgets. If a Phidget is already in use in another program, it will be busy and won't respond to this one.
Still having issues?
Visit the Advanced Troubleshooting Page or contact us (education@phidgets.com).