Errors
In this lesson, you will learn about handling errors that may occur in your code.
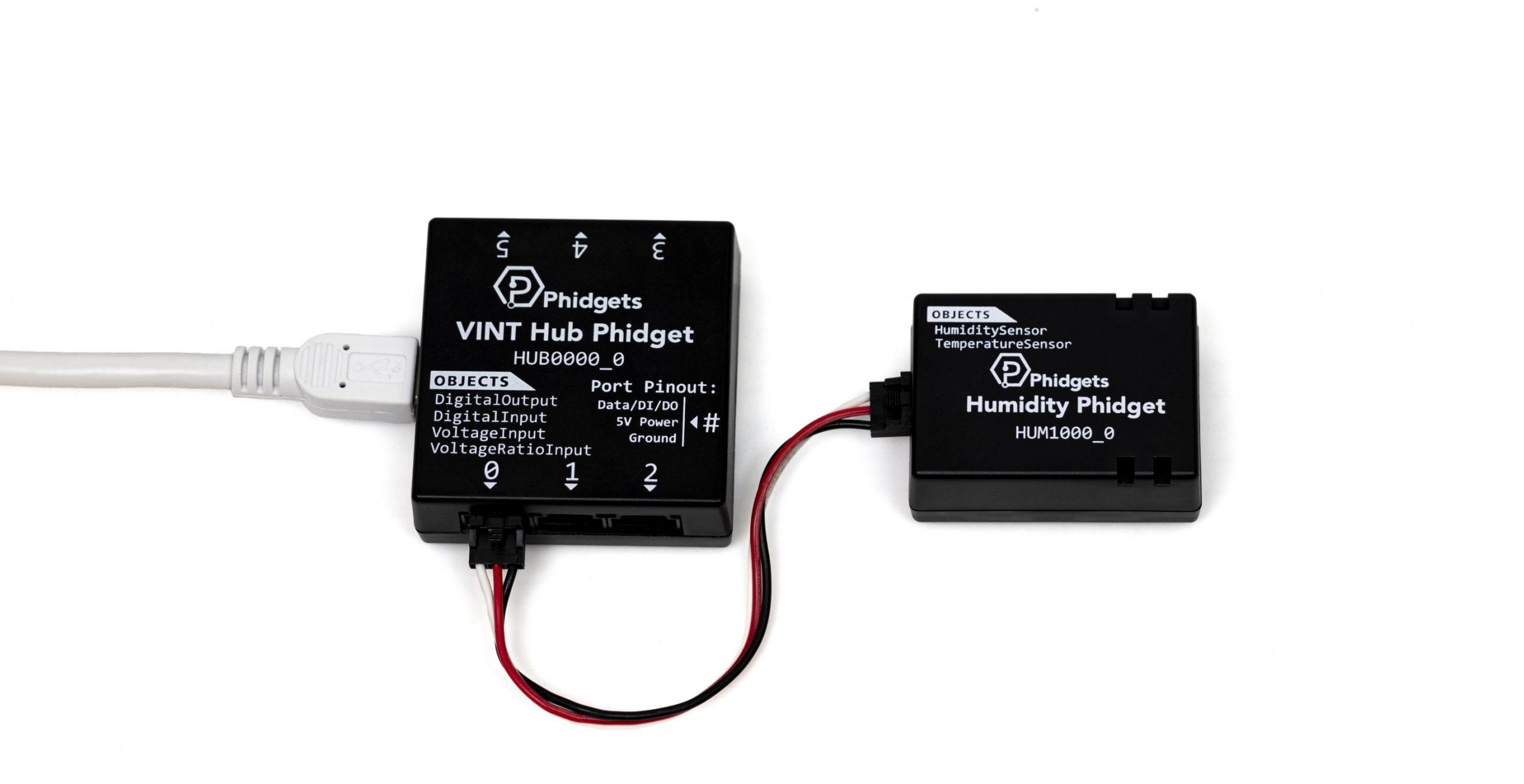
Write code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args){
try{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Use your Phidgets
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
} catch(PhidgetException ex){
//Catch any errors that may occur
System.out.println("Failure: " + ex);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args){
try{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Use your Phidgets
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
} catch(PhidgetException ex){
//Catch any errors that may occur
System.out.println("Failure: " + ex);
}
}
}
Write code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
try:
#Create
temperatureSensor = TemperatureSensor()
#Open
temperatureSensor.openWaitForAttachment(1000)
#Use your Phidgets
print(" Temperature: " + str(temperatureSensor.getTemperature()) + " °C" )
except Exception as ex:
print("Failure: ", ex)
Write code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace GettingStarted
{
class Program
{
public static void Main(string[] args)
{
try
{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.Open(1000);
//Use your Phidgets
System.Console.WriteLine("Temperature: " + temperatureSensor.Temperature + "°C");
} catch (PhidgetException ex)
{
System.Console.WriteLine("Failure: " + ex.Message);
}
}
}
}
Write Code (Swift)
Oops, how did you end up here? There is no Error Events lesson for Swift. Go back to the main tutorial page.
Why Do I Need Error Handling?
Sometimes, a call to a Phidgets function may fail. For example, an error will occur if you try to open a Phidget that is not connected to your computer. It’s a good idea to deal with these errors in your code so that your program doesn’t crash.
How Do I Handle Errors?
If you want to handle errors in your code without causing a crash, surrounding your code with a try-catch or try-except will usually do the trick. This lets the code try to run a piece of code, and then catch the exception if there is a problem.
Do I Have to Handle Errors?
If you have a simple program running in a classroom environment, you may decide not to handle errors and just deal with the occasional error/crash. If you are writing code in a professional setting, you will want to deal with your errors and have your code respond appropriately.
Practice
- Compare the code sample to the code sample in Lesson 4. Note the differences in the code and the program’s output.