Closing Phidgets
In this lesson, you will learn about closing your Phidget.
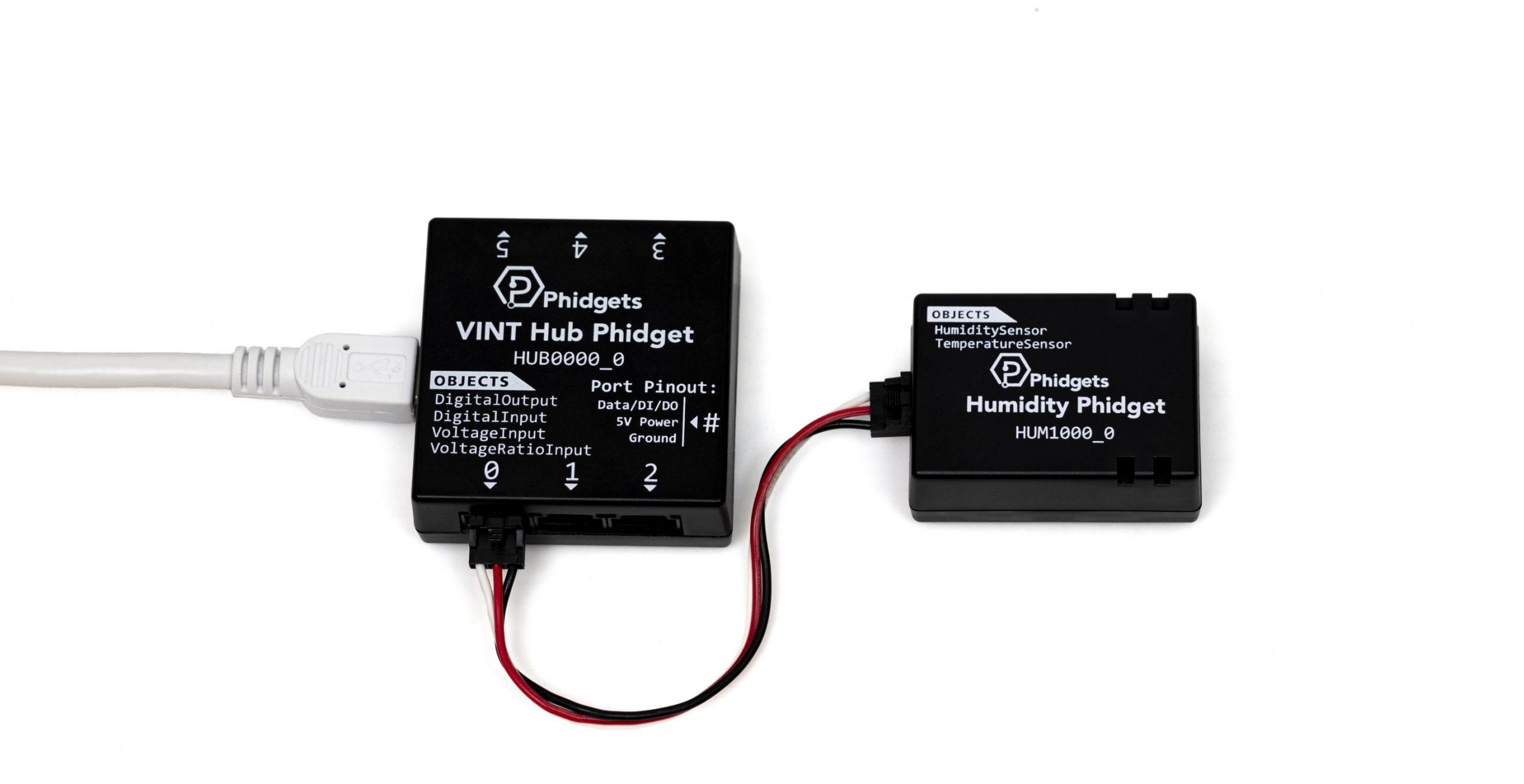
Write Code (Java)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
package gettingstarted;
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Use your Phidgets
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
//Close your Phidgets
temperatureSensor.close();
}
}
//Add Phidgets Library
import com.phidget22.*;
public class GettingStarted {
public static void main(String[] args) throws Exception{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
//Use your Phidgets
System.out.println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
//Close your Phidgets
temperatureSensor.close();
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
TemperatureSensor temperatureSensor;
void setup(){
try{
//Create
temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Temperature: " + temperatureSensor.getTemperature() + " °C" );
//Close your Phidget
temperatureSensor.close();
//Exit program
exit();
}catch(Exception e){
e.printStackTrace();
}
}
Write Code (Python)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.TemperatureSensor import *
#Create
temperatureSensor = TemperatureSensor()
#Open
temperatureSensor.openWaitForAttachment(1000)
#Use your Phidgets
print(" Temperature: " + str(temperatureSensor.getTemperature()) + " °C" )
#Close your Phidgets
temperatureSensor.close()
Write Code (C#)
Copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
//Add Phidgets Library
using Phidget22;
namespace GettingStarted
{
class Program
{
public static void Main(string[] args)
{
//Create
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
temperatureSensor.Open(1000);
//Use your Phidgets
System.Console.WriteLine("Temperature: " + temperatureSensor.Temperature + "°C");
//Close your Phidgets
temperatureSensor.Close();
System.Console.WriteLine("Phidget closed. Press any key to exit.");
System.Console.ReadKey();
}
}
}
Write Code (Swift)
Create a label in your window and copy the code below into the project you created. If you don't have a project or forgot how to create one, revisit the Configure section.
Not your programming language? Set your preferences so we can display relevant code examples
Create a label
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var temperatureLabel: NSTextField!
//Create
let temperatureSensor = TemperatureSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = temperatureSensor.temperatureChange.addHandler(onTemperatureChange)
//Open
try temperatureSensor .open()
}catch{
print(error)
}
}
func onTemperatureChange(sender:TemperatureSensor, temperature: Double){
DispatchQueue.main.async {
//Use your Phidget
self.temperatureLabel.stringValue = String(temperature) + "°C"
}
}
@IBAction func closePhidget(_ sender: NSButton) {
do{
if(try temperatureSensor.getAttached()){
try temperatureSensor.close()
temperatureLabel.stringValue = "Closed"
sender.title = "Open Phidget"
}
else{
try temperatureSensor.open()
sender.title = "Close Phidget"
}
}catch{
print(error)
}
}
}
Run Your Program
You will see the temperature displayed once and then your program will end.
What Does Closing My Phidget Do?
Closing your Phidget breaks the connection between your code and your physical device. This means that after calling close, your object can no longer be used in your code. Closing will also cause the device to return to its default state. For example, if you were powering an LED, the LED would turn off after closing, or, if you were controlling a motor, the motor would stop spinning after calling close.
When Should I Close My Phidget?
For most classroom projects, you won’t have to worry about closing your Phidgets. You may want to consider adding a close statement in the following situations:
- If power is a concern: When Phidgets are in their default state, they use much less power than when they are actively measuring/controlling. If you are using a battery to power your project, you may want to close your Phidget between uses to conserve energy.
- If you want to use your Phidget in multiple programs: A Phidget can only be open in one program at a time (unless you are using networked Phidgets). If you want to share the Phidget between multiple programs, you can close it when you are done.
- If memory is a concern: Creating objects takes up memory, so if you are only using your Phidget for a short period of time, consider closing the Phidget.
Practice
- Try printing temperature from your Phidget after the close call. What error do you get?
- Try calling open again after closing your Phidget. Can you print the temperature again?
Practice
- Try printing temperature from your Phidget after the close call. What error do you get?
- Try calling open again after closing your Phidget. Can you print the temperature again?
Practice
- Try printing temperature from your Phidget after the close call. What error do you get?
- Try calling open again after closing your Phidget. Can you print the temperature again?
Practice
- Are there any new data points after closing the Phidget?
- Try calling open again after closing your Phidget. Can you print the temperature again?