Sound Phidget
The Sound Phidget measures sound pressure level using a small microphone. This allows you to determine how loud an environment is. This Phidget can also provide sound information about 10 individual frequency bands (32Hz, 63Hz, 125Hz, 250Hz, 500Hz, 1kHz, 2kHz, 4kHz, 8kHz, and 16kHz).
The Sound Phidget returns loudness (sound pressure level) in decibels (symbol: dB). A typical vacuum will be around 80dB, whereas a typical Library will be around 40bB.
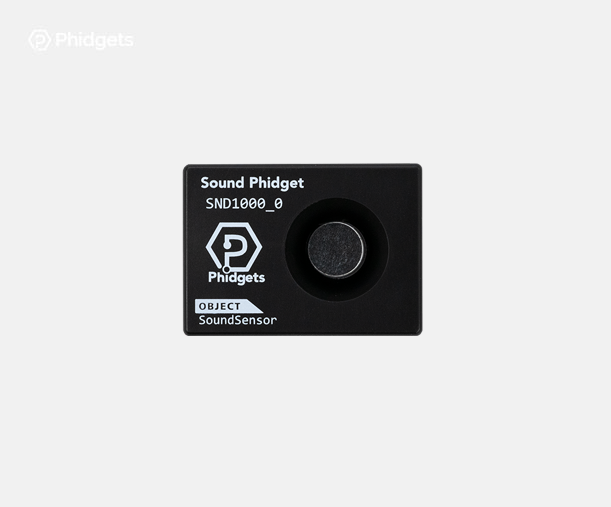
Code (Java)
Create a file called Sonar and insert the following code. Run your code. Clap your hands to see a dB change.
Not your programming language? Set my language and IDE.
package sound;
//Add Phidgets Library
import com.phidget22.*;
public class Sound {
public static void main(String[] args) throws Exception {
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Sound pressure level: " + soundSensor.getdB() + " dB");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class Sound {
public static void main(String[] args) throws Exception {
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
//Use your Phidgets
while (true) {
System.out.println("Sound pressure level: " + soundSensor.getdB() + " dB");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
SoundSensor soundSensor;
void setup(){
try{
//Create
soundSensor = new SoundSensor();
//Open
soundSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Sound pressure level: " + soundSensor.getdB() + " dB");
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Sonar and insert the following code. Run your code. Clap your hands to see a dB change.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.SoundSensor import *
#Required for sleep statement
import time
#Create
soundSensor = SoundSensor()
#Open
soundSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Sound pressure level: " + str(soundSensor.getdB()) + " dB")
time.sleep(0.25)
Code (C#)
Create a file called Sonar and insert the following code. Run your code. Clap your hands to see a dB change.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Sound
{
class Program
{
static void Main(string[] args)
{
//Create
SoundSensor soundSensor = new SoundSensor();
//Open
soundSensor.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("Sound pressure level: " + soundSensor.dB + " dB");
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called Sonar and insert the following code. Run your code. Clap your hands to see a dB change.
Not your programming language? Set my language and IDE.
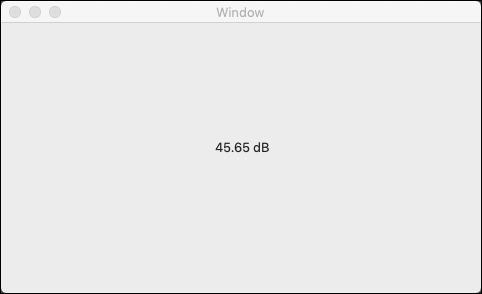
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let soundSensor = SoundSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = soundSensor.SPLChange.addHandler(onSPLChange)
//Open
try soundSensor.open()
}catch{
print(error)
}
}
func onSPLChange(sender:SoundSensor, data: (dB: Double, dBA: Double, dBC: Double, octaves: [Double])){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = String(data.dB) + " dB"
}
}
}
Applications
Sound sensors are used in a wide variety of applications:
- Sound sensors are used in manufacturing, construction and other loud environments to determine what level of personal protective equipment (e.g. earplugs) is required.
- Security systems use sound sensors to better understand the environment they are monitoring.
- Sound engineers use sound sensors to balance audio or measure sound levels in auditoriums.
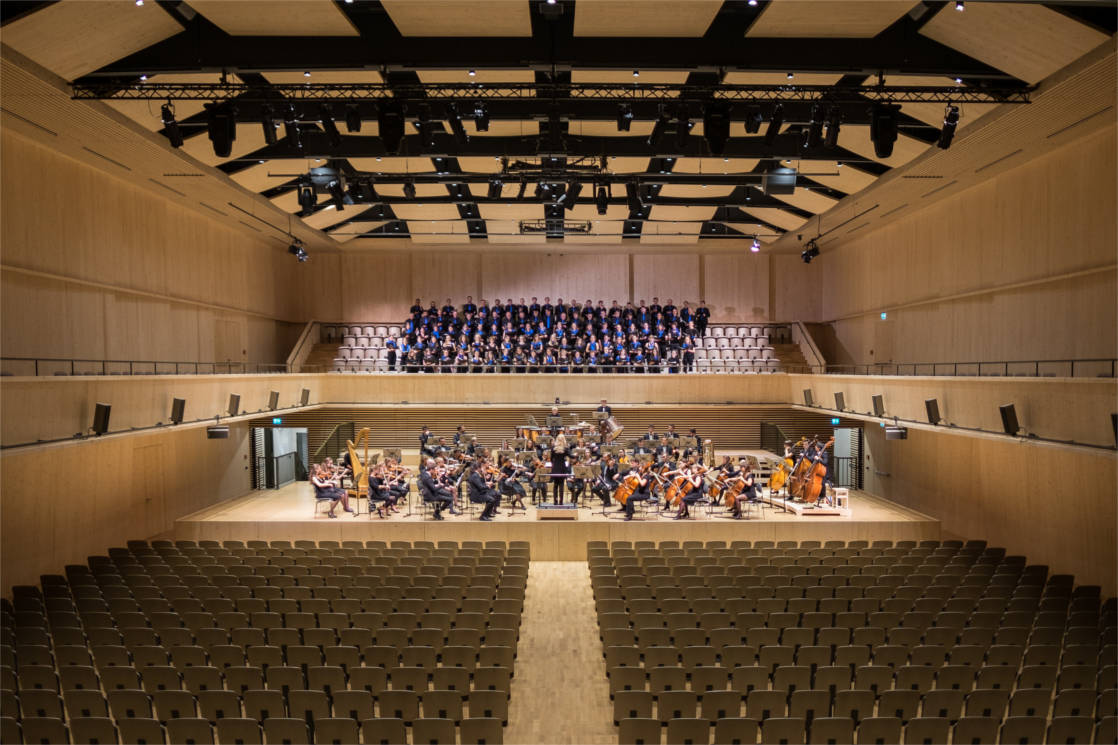
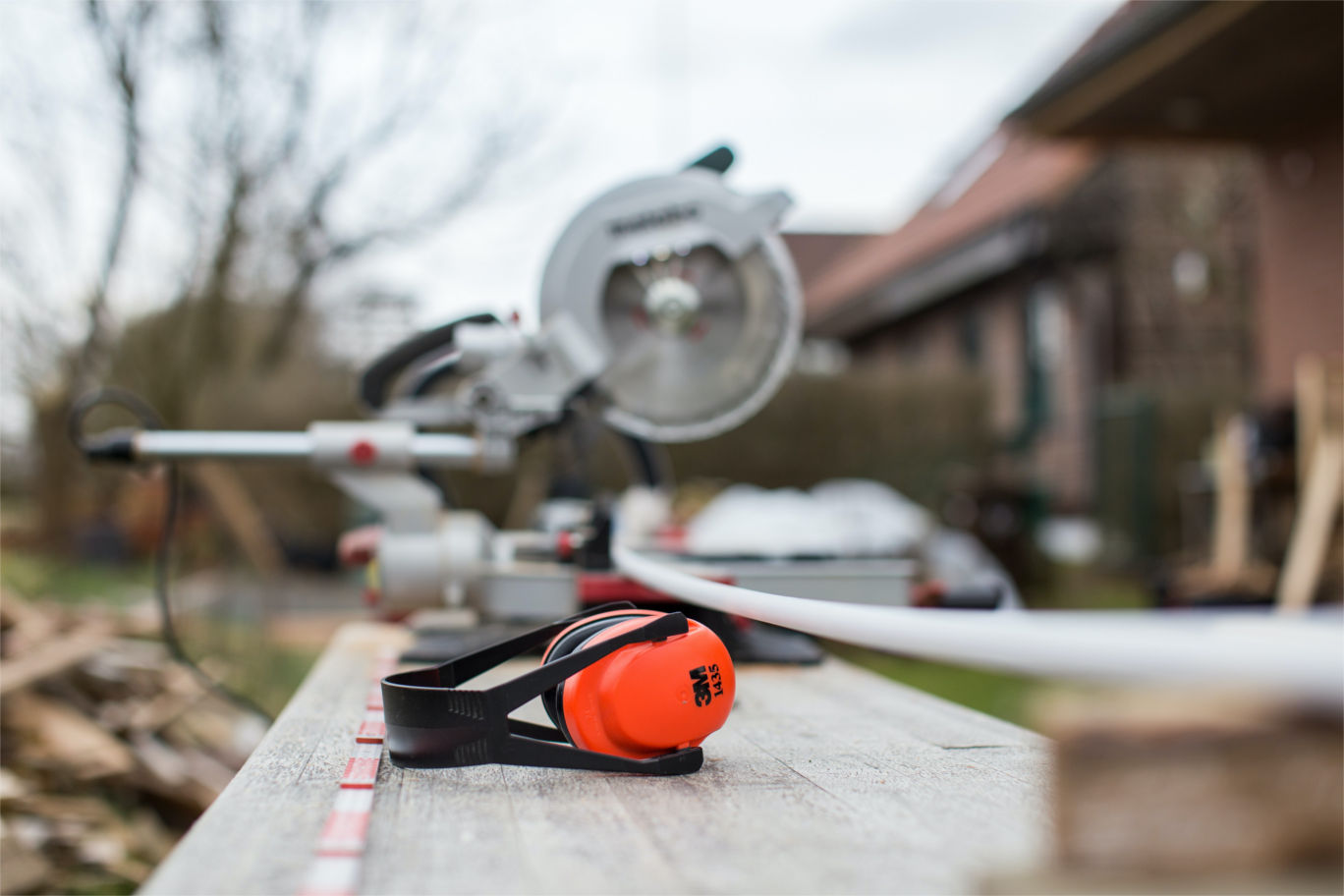
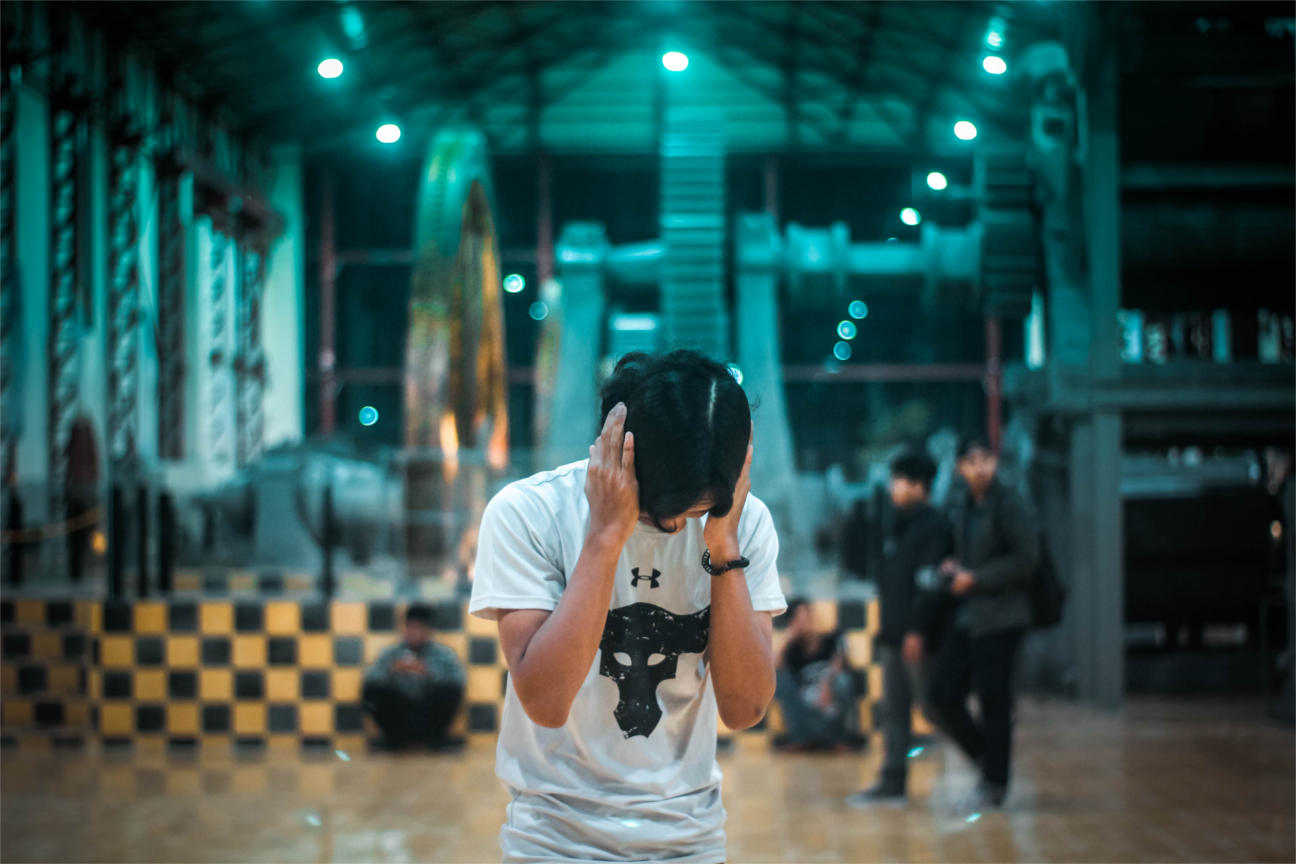
Practice
Using your Getting Started Kit and Sound Phidget, you can simulate a Clapper light system.
- Replace your Humidity Phidget with the Sound Phidget.
- Write a program that uses the Sound Phidget to detect 2 consecutive claps. (You will need to determine how many decibels a clap is).
Check out the advanced lesson Using the Sensor API before you use the API for the first time.