RFID Phidget
RFID stands for Radio Frequency Identification. The RFID Phidget allows you to read from and write to RFID tags. RFID tags store small amounts of data. Data is wirelessly transmitted to the RFID reader when a tag comes within range of the RFID reader.
The RFID is unique as it does not require a VINT Hub.
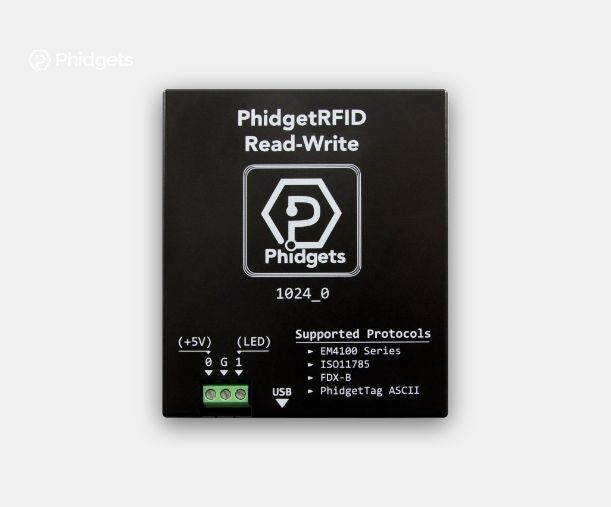
Setup
Before you do any coding you will have to attach your RFID Phidget to your computer through a USB port:
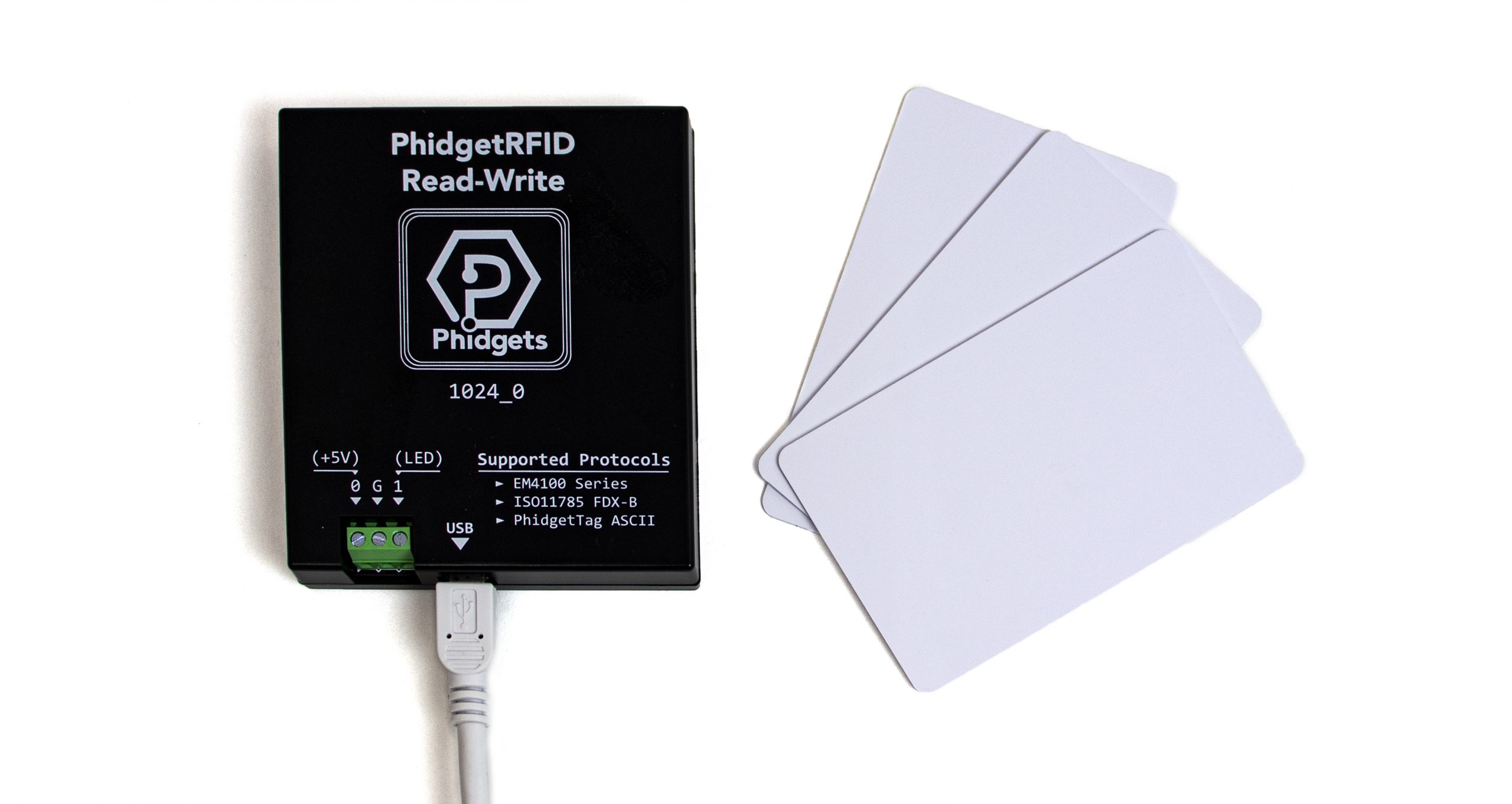
Code (Java)
Create a file called RFIDPhidget and insert the following code. Connect your RFID Phidget to your Computer, you do not need a VINT Hub. Run your code. Tap your RFID Tags.
Not your programming language? Set my language and IDE.
package rfidphidget;
//Add Phidgets Library
import com.phidget22.*;
public class RFIDPhidget {
public static void main(String[] args) throws Exception {
//Create
RFID rfid = new RFID();
//Open
rfid.open(1000);
//Use your Phidget
while (true) {
if (rfid.getTagPresent()) {
System.out.println(rfid.getLastTag().tagString);
}
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class RFIDPhidget {
public static void main(String[] args) throws Exception {
//Create
RFID rfid = new RFID();
//Open
rfid.open(1000);
//Use your Phidget
while (true) {
if (rfid.getTagPresent()) {
System.out.println(rfid.getLastTag().tagString);
}
Thread.sleep(150);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
RFID rfid;
void setup(){
try{
//Create
rfid = new RFID();
//Open
rfid.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
if (rfid.getTagPresent()) {
System.out.println(rfid.getLastTag().tagString);
}
delay(500);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called RFID and insert the following code. Connect your RFID Phidget to your Computer, you do not need a VINT Hub. Run your code. Tap your RFID Tags.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.RFID import *
#Required for sleep statement
import time
#Create
rfid = RFID()
#Open
rfid.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
if(rfid.getTagPresent()):
print(rfid.getLastTag()[0])
time.sleep(0.15)
Code (C#)
Create a file called RFIDPhidget and insert the following code. Connect your RFID Phidget to your Computer, you do not need a VINT Hub. Run your code. Tap your RFID Tags.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace RFID{
class Program{
static void Main(string[] args){
//Create
RFID rfid = new RFID();
//Open
rfid.Open(1000);
//Use your Phidgets
while (true){
if (rfid.TagPresent){
System.Console.WriteLine(rfid.GetLastTag().TagString);
}
System.Threading.Thread.Sleep(150);
}
}
}
}
Code (Swift)
Create a file called RFID and insert the following code. Connect your RFID Phidget to your Computer, you do not need a VINT Hub. Run your code. Tap your RFID Tags.
Not your programming language? Set my language and IDE.
You will need to add a Label.
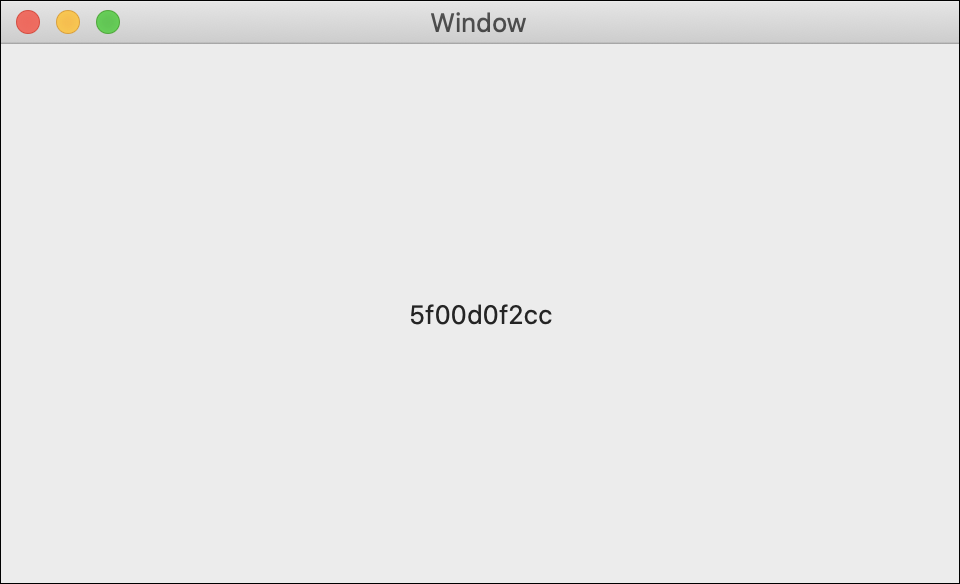
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let rfid = RFID()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to events
let _ = rfid.tag.addHandler(onTagFound)
let _ = rfid.tagLost.addHandler(onTagLost)
//Open
try rfid.open()
}catch{
print(error)
}
}
func onTagFound(sender:RFID, data: (tag:String, proto:RFIDProtocol)){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = data.tag
}
}
func onTagLost(sender:RFID, data: (tag:String, proto:RFIDProtocol)){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = "No Tag Present"
}
}
}
Applications
If you have ever tapped a plastic card against a door to gain entry, you’ve used RFID technology. RFID tags and readers are in use in a wide variety of industries and applications, from inventory management in large warehouses to animal identification in agriculture to tracking machines on a factory floor.
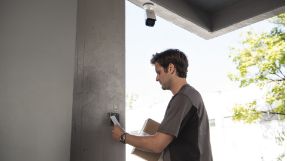
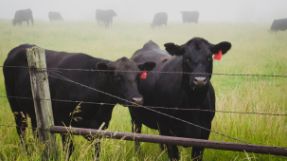
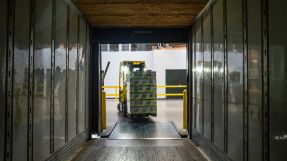
Practice
Using your RFID Phidget and the Getting Started Kit, create an indicator similar to those on door access systems all over the world.
- When a tag is detected, turn on the green LED.
- When there is no tag, turn on the red LED.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.