pH Phidget
The pH Phidget measures the pH of a liquid. pH is a measure of how acidic or basic (alkaline) a water-based solution is.
The pH scale runs from 0 (very acidic) to 14 (very basic)—7 being neutral. For example, vinegar is acidic, with a pH generally around 2.4. Baking soda dissolved into water will have a pH of approximately 9.3.
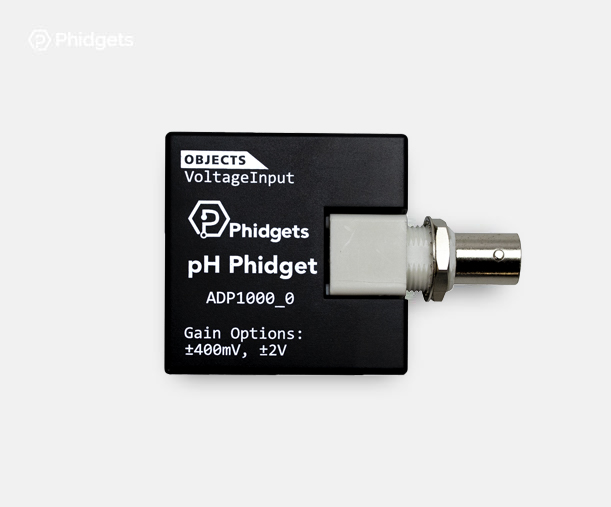
Code (Java)
Create a file called PH and insert the following code. Run your code. Place your electrode in a glass of water to see the pH.
Not your programming language? Set my language and IDE.
package ph;
//Add Phidgets Library
import com.phidget22.*;
public class PH{
public static void main(String[] args) throws Exception{
//Create
PHSensor phSensor = new PHSensor();
//Open
phSensor.open(1000);
//Use your Phidgets
while(true){
System.out.println("pH: " + phSensor.getPH()+ " pH");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class PH{
public static void main(String[] args) throws Exception{
//Create
PHSensor phSensor = new PHSensor();
//Open
phSensor.open(1000);
//Use your Phidgets
while(true){
System.out.println("pH: " + phSensor.getPH()+ " pH");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
PHSensor phSensor;
void setup(){
try{
//Create
phSensor = new PHSensor();
//Open
phSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("pH: " + phSensor.getPH()+ " pH");
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called PH and insert the following code. Run your code. Place your electrode in a glass of water to see the pH.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.PHSensor import *
#Required for sleep statement
import time
#Create
phSensor = PHSensor()
#Open
phSensor.openWaitForAttachment(1000)
#Use your Phidgets
while(True):
print("PH: " + str(phSensor.getPH()) + " pH")
time.sleep(0.25)
Code (C#)
Create a file called PH and insert the following code. Run your code. Place your electrode in a glass of water to see the pH.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace PH{
class Program{
static void Main(string[] args){
//Create
PHSensor phSensor = new PHSensor();
//Open
phSensor.Open(1000);
//Use your Phidgets
while (true)
{
System.Console.WriteLine("pH: " + phSensor.PH + " pH");
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called PH and insert the following code. Run your code. Place your electrode in a glass of water to see the pH.
Not your programming language? Set my language and IDE.
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let pHSensor = PHSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = pHSensor.PHChange.addHandler(onPHChange)
//Open
try pHSensor.open()
}catch{
print(error)
}
}
func onPHChange(sender:PHSensor, pH:Double){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = String(pH) + " pH"
}
}
}
Electrode Information - Storage/Initial Use
When not in use, store your electrode in the buffer solution.
Note: for best results, soak the electrode in the buffer solution overnight prior to first use.
Electrode Information - Important Notes
- Never wipe an electrode. Blot the end of the electrode with a lint-free paper to remove excess solution.
- Never directly touch the glass membrane of the electrode with your fingers.
- If possible, rinse electrode between samples with distilled or deionized water for best results.
Electrode Information - Advanced Users
If you are an Advanced User and you want to obtain the most accurate results, please review this guide.
Applications
pH monitoring has several scientific and industrial applications. pH sensors monitor titration experiments or other chemistry applications. Fermented foods use pH monitoring to ensure consistency and proper fermentation. Farms use pH sensors to watch the pH of the soil to ensure maximum crop success.
You may notice some soaps and skincare products list the pH. The pH of a product can influence the dryness and bacteria found on the skin.
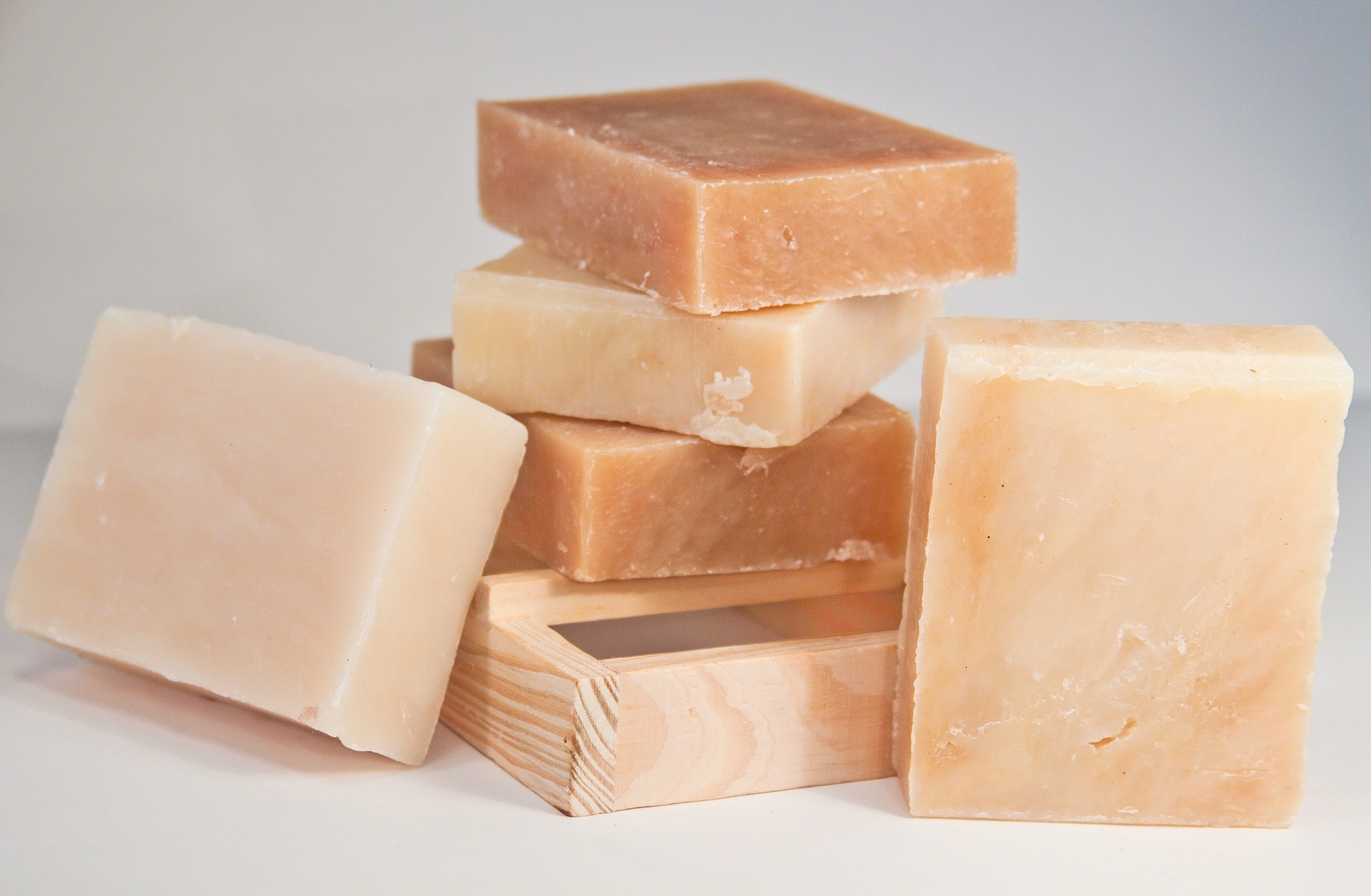
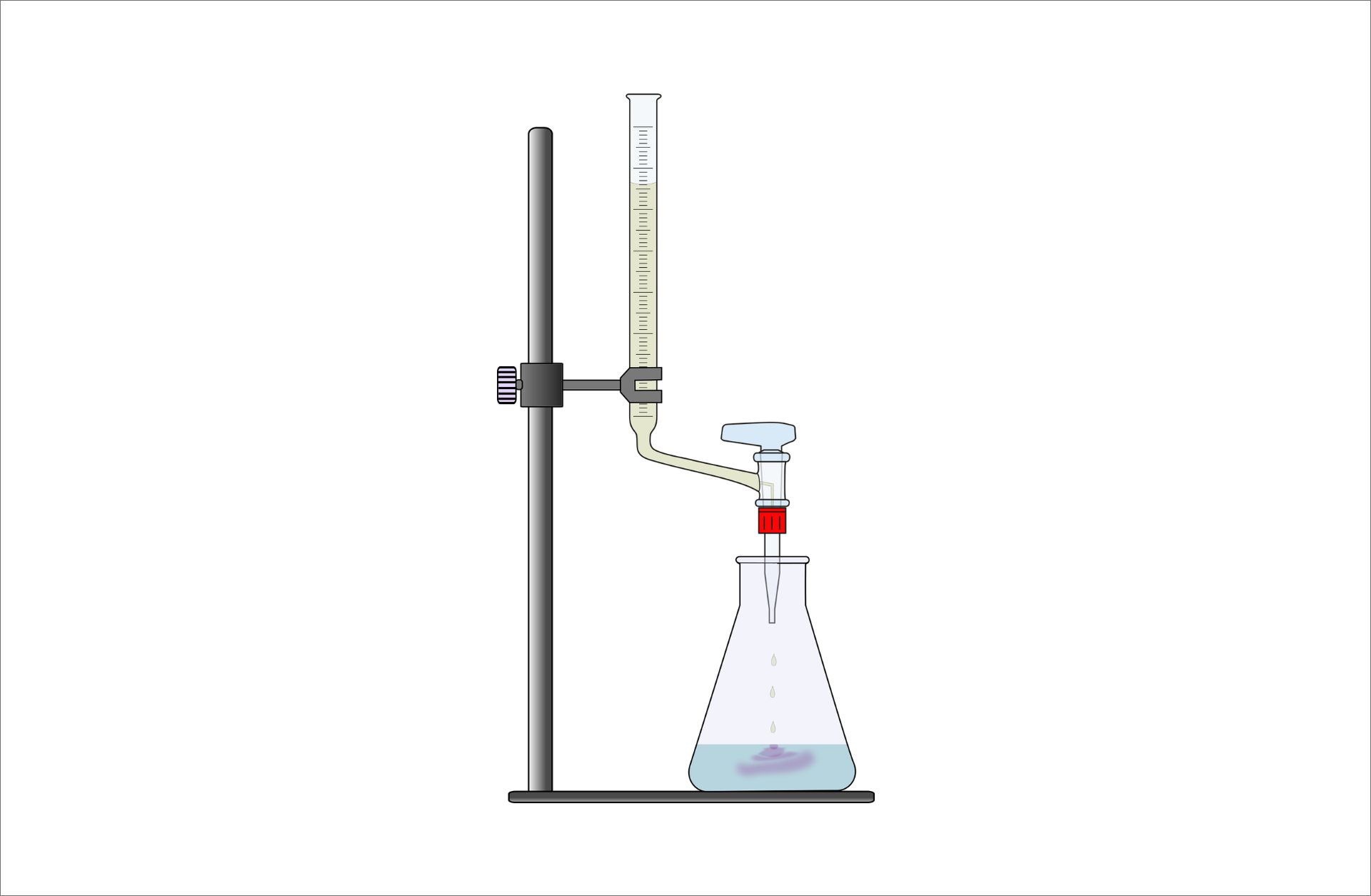
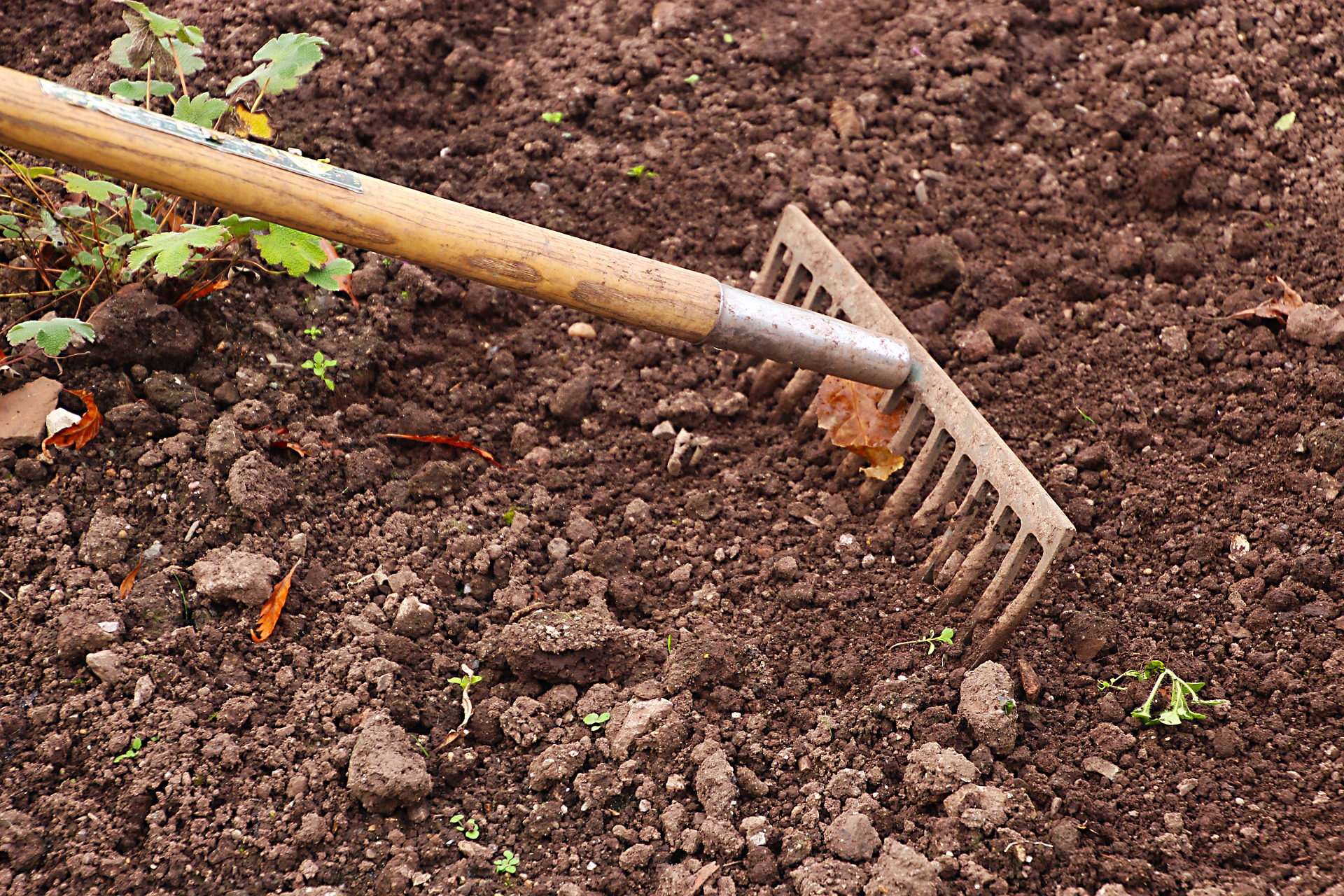
Practice
You can use your Getting Started Kit and pH Phidget to create a pH indicator.
- Attach the pH Phidget to the VINT Hub in your Getting Started Kit.
- Write a program that uses your LEDs to indicate if a solution is acidic or basic and test it with some vinegar or a baking soda solution.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.