Light Phidget
The Light Phidget measures brightness. The sensor in the Light Phidget closely matches the human eye's sensitivity.
The Light Phidget returns brightness (called illuminance) in lux (symbol: lx). Lux is a measure of how much light there is per square meter. A typical classroom will be around 250 lx.
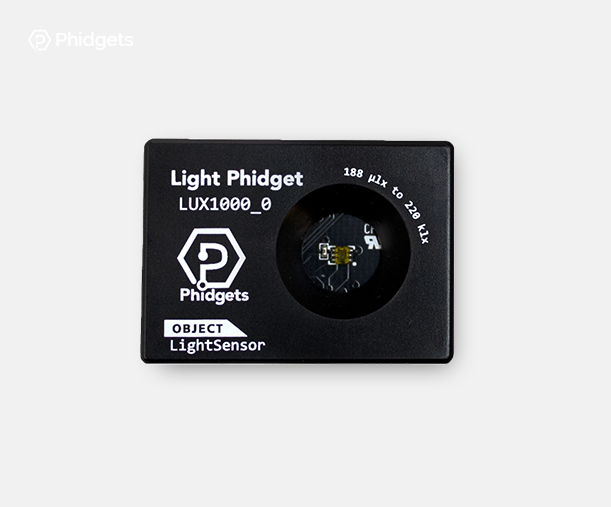
Code (Java)
Create a file called Light and add the following code. Run your code to see the illuminance of your classroom. Try covering the sensor or shining a light on the sensor to see the changes in output.
Not your programming language? Set my language and IDE.
package light;
//Add Phidgets Library
import com.phidget22.*;
public class Light{
public static void main(String[] args) throws Exception{
//Create
LightSensor lightSensor = new LightSensor();
//Open
lightSensor.open(1000);
//Use your Phidgets
while(true){
System.out.println("Illuminance: " + lightSensor.getIlluminance() + " lx");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class Light{
public static void main(String[] args) throws Exception{
//Create
LightSensor lightSensor = new LightSensor();
//Open
lightSensor.open(1000);
//Use your Phidgets
while(true){
System.out.println("Illuminance: " + lightSensor.getIlluminance() + " lx");
Thread.sleep(250);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
LightSensor lightSensor;
void setup(){
try{
//Create
lightSensor = new LightSensor();
//Open
lightSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Illuminance: " + lightSensor.getIlluminance() + " lx");
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Light and add the following code. Run your code to see the illuminance of your classroom. Try covering the sensor or shining a light on the sensor to see the changes in output.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.LightSensor import *
#Required for sleep statement
import time
#Create
lightSensor = LightSensor()
#Open
lightSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Illuminance: " + str(lightSensor.getIlluminance()) + " lx")
time.sleep(0.25)
Code (C#)
Create a file called Light and add the following code. Run your code to see the illuminance of your classroom. Try covering the sensor or shining a light on the sensor to see the changes in output.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Light{
class Program{
static void Main(string[] args){
//Create
LightSensor lightSensor = new LightSensor();
//Open
lightSensor.Open(1000);
//Use your Phidgets
while (true){
System.Console.WriteLine("Illuminance: " + lightSensor.Illuminance + " lx");
System.Threading.Thread.Sleep(250);
}
}
}
}
Code (Swift)
Create a file called Light and add the following code. Run your code to see the illuminance of your classroom. Try covering the sensor or shining a light on the sensor to see the changes in output.
Not your programming language? Set my language and IDE.
You will need to add a Label.
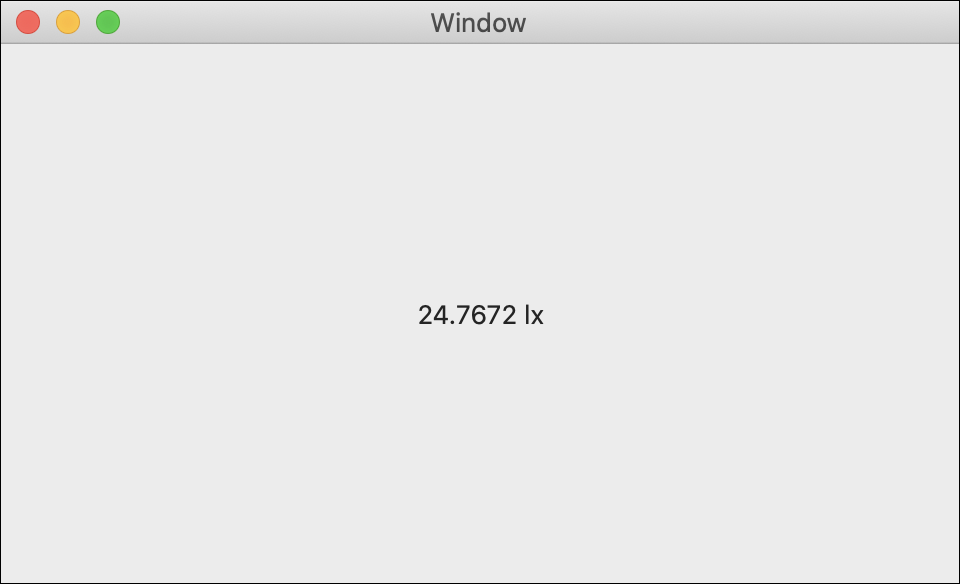
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let lightSensor = LightSensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = lightSensor.illuminanceChange.addHandler(onIlluminanceChange)
//Open
try lightSensor.open()
}catch{
print(error)
}
}
func onIlluminanceChange(sender:LightSensor, illuminance:Double){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = String(illuminance) + " lux"
}
}
}
Applications
If you have ever noticed that your phone or tablet gets brighter or dimmer depending on your environment, you have witnessed a light sensor in action!
- Modern cars use light sensors to automatically turn their headlights on or off.
- Many outdoor lamps come on at the perfect time due to light sensors.
- Greenhouses and other similar facilities use light sensors to monitor and maintain their plantlife.
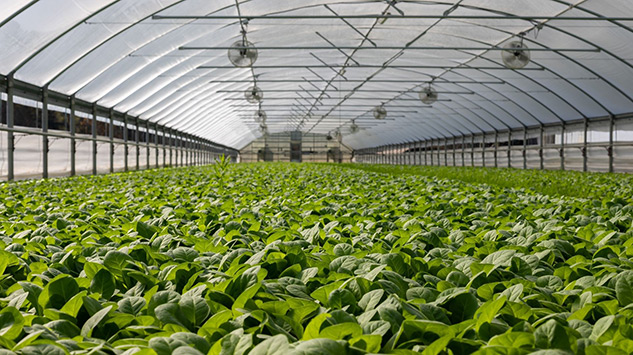
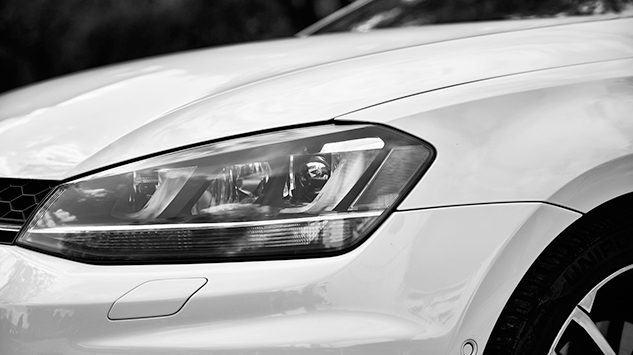
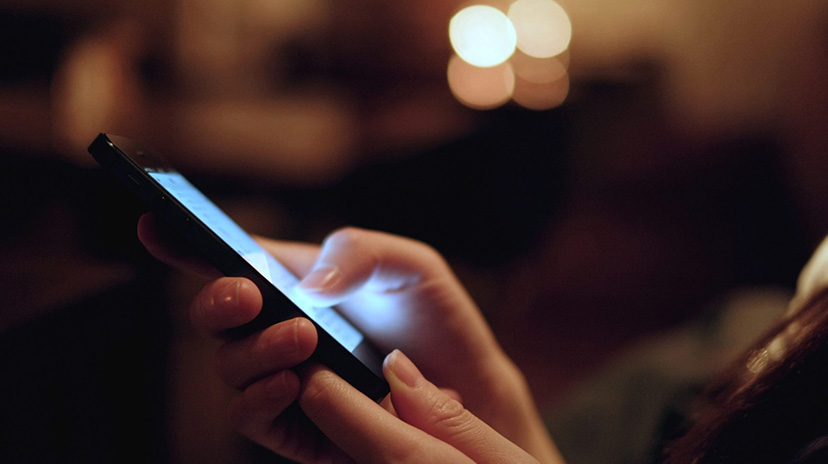
Practice
Using your Getting Started Kit and Light Phidget, you can simulate a shipping container security system.
- Replace your Humidity Phidget with the Light Phidget.
- Place the Light Phidget inside the box.
- Write a program that uses the Light Phidget to let you know when the box is open or closed.
- Turn on the green LED when the box is closed and turn the Red LED on when the box is open.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.