Humidity Phidget
The Humidity Phidget measures relative humidity. Relative humidity lets you know how much moisture is in the air. The Phidget returns humidity in a percentage. Between 30% and 50% relative humidity is considered comfortable.
Note: The Humidity Phidget also has a temperature sensor built-in, measuring in degrees Celsius.
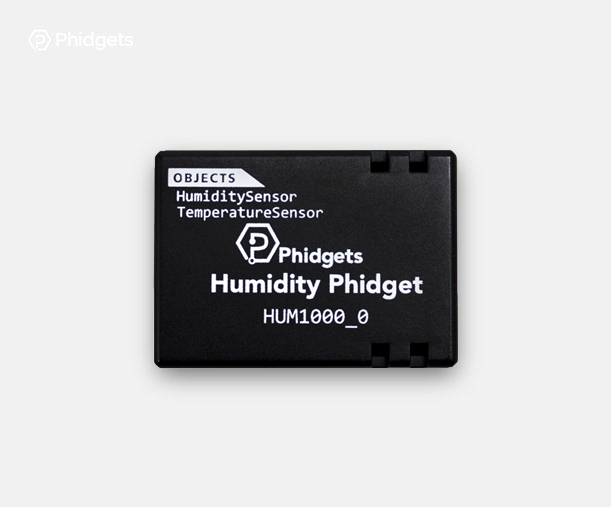
Code (Java)
Create a file called Humidity and insert the following code. Run your code. Place your hand over the sensor to see the humidity change.
Not your programming language? Set my language and IDE.
package humidity;
//Add Phidgets Library
import com.phidget22.*;
public class Humidity{
public static void main(String[] args) throws Exception{
//Create
HumiditySensor humiditySensor = new HumiditySensor();
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
humiditySensor.open(1000);
temperatureSensor.open(1000);
//Use your Phidgets
while(true){
System.out.println("Humidity: " + humiditySensor.getHumidity() +" %RH, Temperature: " + temperatureSensor.getTemperature() + " ℃" );
Thread.sleep(500);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
public class Humidity{
public static void main(String[] args) throws Exception{
//Create
HumiditySensor humiditySensor = new HumiditySensor();
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
humiditySensor.open(1000);
temperatureSensor.open(1000);
//Use your Phidgets
while(true){
System.out.println("Humidity: " + humiditySensor.getHumidity() +" %RH, Temperature: " + temperatureSensor.getTemperature() + " ℃" );
Thread.sleep(500);
}
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
HumiditySensor humiditySensor;
TemperatureSensor temperatureSensor;
void setup(){
try{
//Create
humiditySensor = new HumiditySensor();
temperatureSensor = new TemperatureSensor();
//Open
humiditySensor.open(1000);
temperatureSensor.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
println("Humidity: " + humiditySensor.getHumidity() +" %RH, Temperature: " + temperatureSensor.getTemperature() + " °C" );
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called Humidity and insert the following code. Run your code. Place your hand over the sensor to see the humidity change.
Not your programming language? Set my language and IDE.
#Add Phidgets library
from Phidget22.Phidget import *
from Phidget22.Devices.HumiditySensor import *
from Phidget22.Devices.TemperatureSensor import *
#Required for sleep statement
import time
#Create
humiditySensor = HumiditySensor()
temperatureSensor = TemperatureSensor()
#Open
humiditySensor.openWaitForAttachment(1000)
temperatureSensor.openWaitForAttachment(1000)
#Use your Phidgets
while (True):
print("Humidity: " + str(humiditySensor.getHumidity()) + " %RH")
print("Temperature: " + str(temperatureSensor.getTemperature()) + "°C\n")
time.sleep(0.5)
Code (C#)
Create a file called Humidity and insert the following code. Run your code. Place your hand over the sensor to see the humidity change.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace Humidity{
class Program{
static void Main(string[] args) {
//Create
HumiditySensor humiditySensor = new HumiditySensor();
TemperatureSensor temperatureSensor = new TemperatureSensor();
//Open
humiditySensor.Open(1000);
temperatureSensor.Open(1000);
//Use your Phidgets
while (true){
System.Console.WriteLine("Humidity: " + humiditySensor.Humidity + " %RH, Temperature: " + temperatureSensor.Temperature + "°C");
System.Threading.Thread.Sleep(500);
}
}
}
}
Code (Swift)
Create a file called Humidity and insert the following code. Run your code. Place your hand over the sensor to see the humidity change.
Not your programming language? Set my language and IDE.
You will need to add a Label.
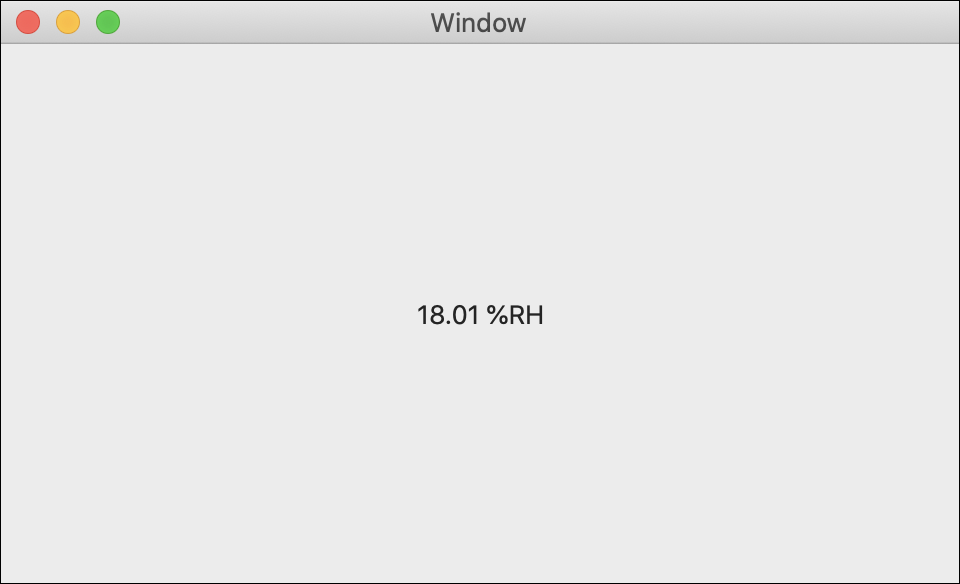
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let humiditySensor = HumiditySensor()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Subscribe to event
let _ = humiditySensor.humidityChange.addHandler(onHumidityChange)
//Open
try humiditySensor.open()
}catch{
print(error)
}
}
func onHumidityChange(sender:HumiditySensor, humidity:Double){
DispatchQueue.main.async {
//Use information from your Phidget to change label
self.sensorLabel.stringValue = String(humidity) + " %RH"
}
}
}
Applications
Humidity sensors are used in climate control systems to monitor and maintain temperature and humidity levels. Refrigeration, cargo storage, electronics manufacturers, museums, baby incubators, and other hospital applications all require stable humidity. Weather monitoring also uses humidity sensors. Higher humidity means a greater chance of precipitation.
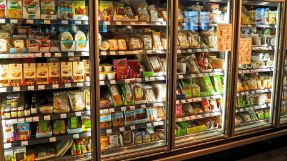
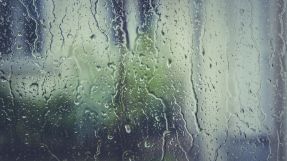
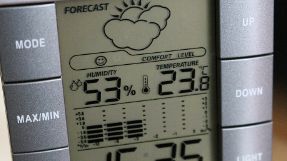
Practice
You have already used the Humidity Phidget with the Getting Started Kit. For more practice, you can create a temperature or humidity guessing game. Print a target temperature or humidity to your user and ask if they think the current temperature/humidity is higher or lower. Your user will answer higher or lower by pressing the green button or the red button.
After your user answers, use the LED to inform them if they are right (green) or wrong (red) and print the temperature.
Check out the advanced lesson Using the Sensor API before you use the API for the first time.