Graphic LCD Phidget
The Graphic LCD Phidget lets you display text and simple images.
It has a screen that is 128 pixels wide and 64 pixels tall. You can adjust the backlight brightness and the pixel contrast. There are also methods available to draw single pixels, straight lines and rectangles.
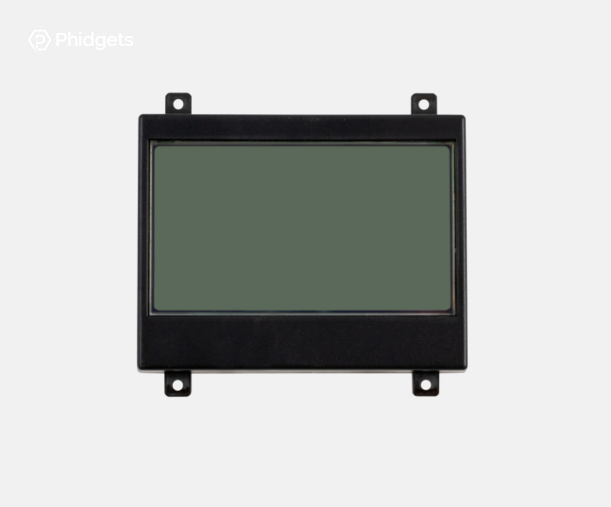
Code (Java)
Create a file called GraphicLCD and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
package graphiclcd;
//Add Phidgets Library
import com.phidget22.*;
public class GraphicLCD{
public static void main(String[] args) throws Exception{
//Create
LCD lcd = new LCD();
//Open
lcd.open(1000);
//Use your Phidgets
lcd.writeText(LCDFont.DIMENSIONS_6X12, 0, 0, "Hello, World!");
lcd.flush();
}
}
//Add Phidgets Library
import com.phidget22.*;
public class GraphicLCD{
public static void main(String[] args) throws Exception{
//Create
LCD lcd = new LCD();
//Open
lcd.open(1000);
//Use your Phidgets
lcd.writeText(LCDFont.DIMENSIONS_6X12, 0, 0, "Hello, World!");
lcd.flush();
}
}
//Add Phidgets Library
import com.phidget22.*;
//Define
LCD lcd;
void setup(){
try{
//Create
lcd = new LCD();
//Open
lcd.open(1000);
}catch(Exception e){
e.printStackTrace();
}
}
void draw(){
try{
//Use your Phidgets
lcd.writeText(LCDFont.DIMENSIONS_6X12, 0, 0, "Hello, World!");
lcd.flush();
delay(250);
}catch(Exception e){
e.printStackTrace();
}
}
Code (Python)
Create a file called GraphicLCD and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
#Add Phidgets Library
from Phidget22.Phidget import *
from Phidget22.Devices.LCD import *
import time
#Create
lcd = LCD()
#Open
lcd.openWaitForAttachment(1000)
#Use your Phidgets
lcd.writeText(LCDFont.FONT_6x12, 0, 0, "Hello, World!")
lcd.flush()
Code (C#)
Create a file called GraphicLCD and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
//Add Phidgets Library
using Phidget22;
namespace GraphicLCD
{
class Program
{
static void Main(string[] args)
{
//Create
LCD lcd = new LCD();
//Open
lcd.Open(1000);
//Use your Phidgets
lcd.WriteText(LCDFont.Dimensions_6x12, 0, 0, "Hello, World!");
lcd.Flush();
//Prevent console from closing immediately
System.Threading.Thread.Sleep(5000);
}
}
}
Code (Swift)
Create a file called GraphicLCD and insert the following code. Run your code.
Not your programming language? Set my language and IDE.
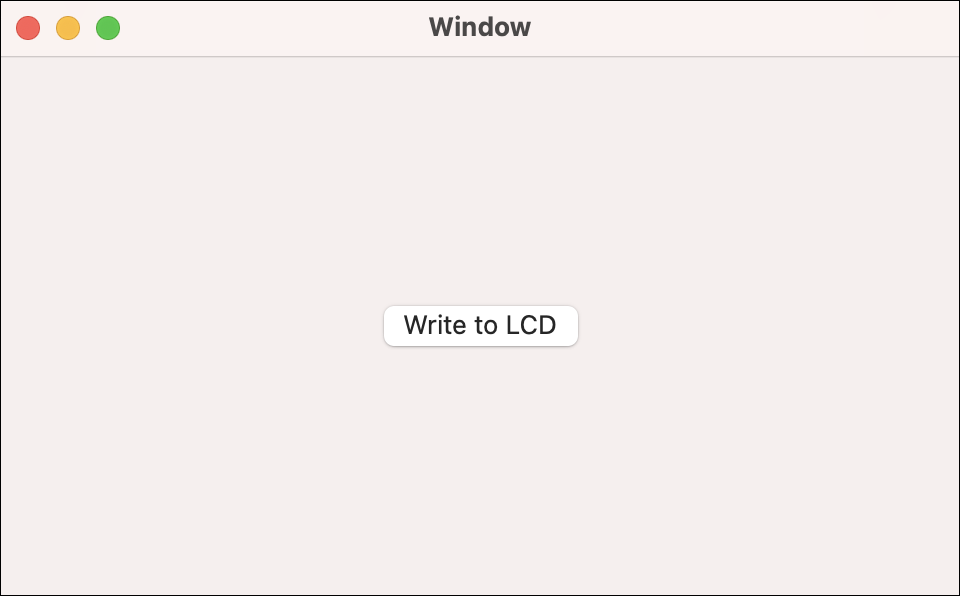
import Cocoa
//Add Phidgets Library
import Phidget22Swift
class ViewController: NSViewController {
@IBOutlet weak var sensorLabel: NSTextField!
//Create
let lcd = LCD()
override func viewDidLoad() {
super.viewDidLoad()
do{
//Open
try lcd.open()
}catch{
print(error)
}
}
@IBAction func writeToLCD(_ sender: Any) {
do{
try lcd.writeText(font: LCDFont.dimensions_6x12,xPosition: 0,yPosition: 0,text: "Hello, World!")
try lcd.flush()
}catch{
print(error)
}
}
}
Applications
LCD technology is used in an extremely wide range of applications. From televisions and monitors to cameras and watches, you have likely interacted with many LCD screens!
The Graphic LCD is also a great way to display information if no output device is available. For example, if you are monitoring a greenhouse with a Raspbery Pi, you could use the Graphic LCD to display relevant information to users.
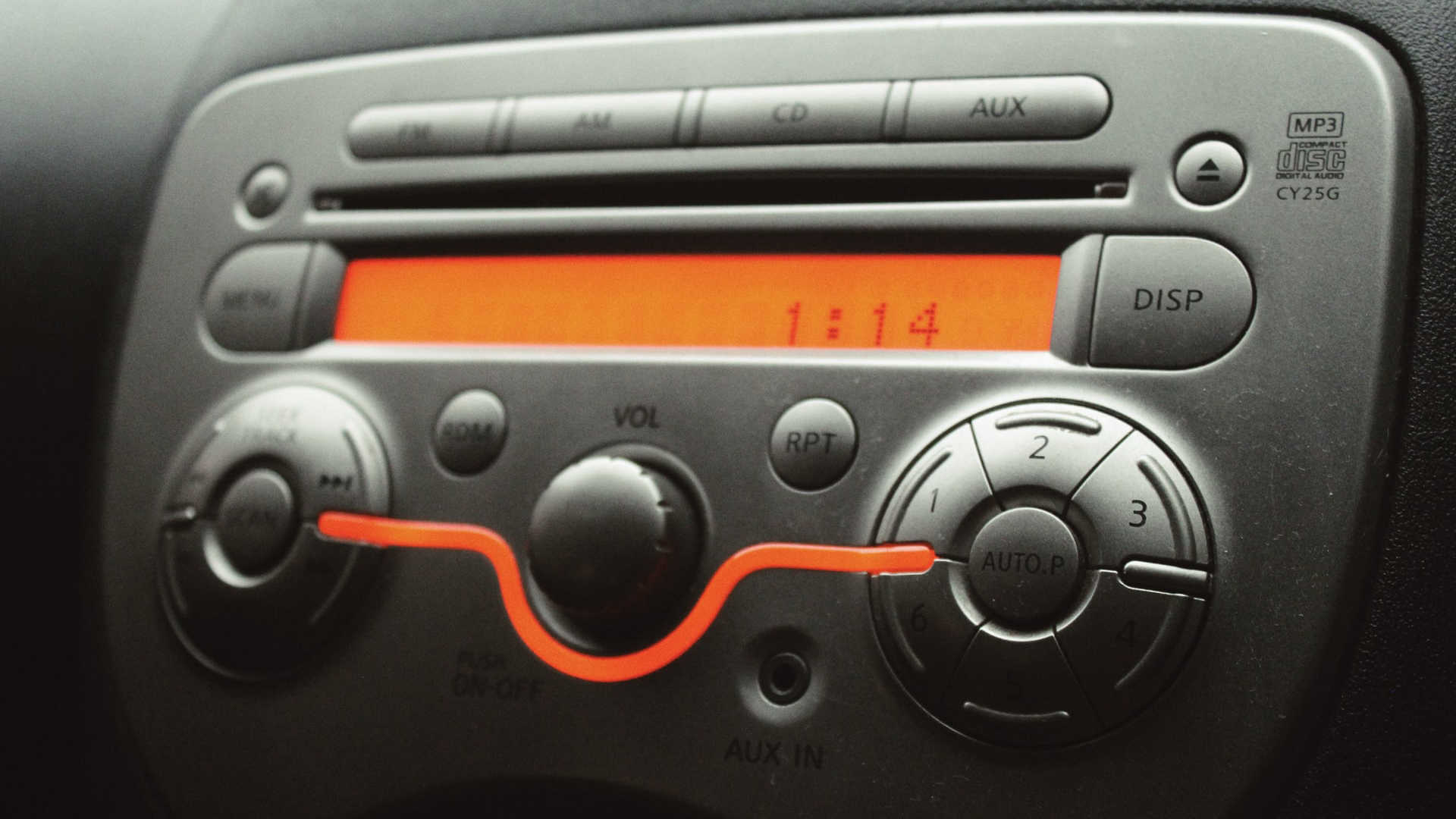
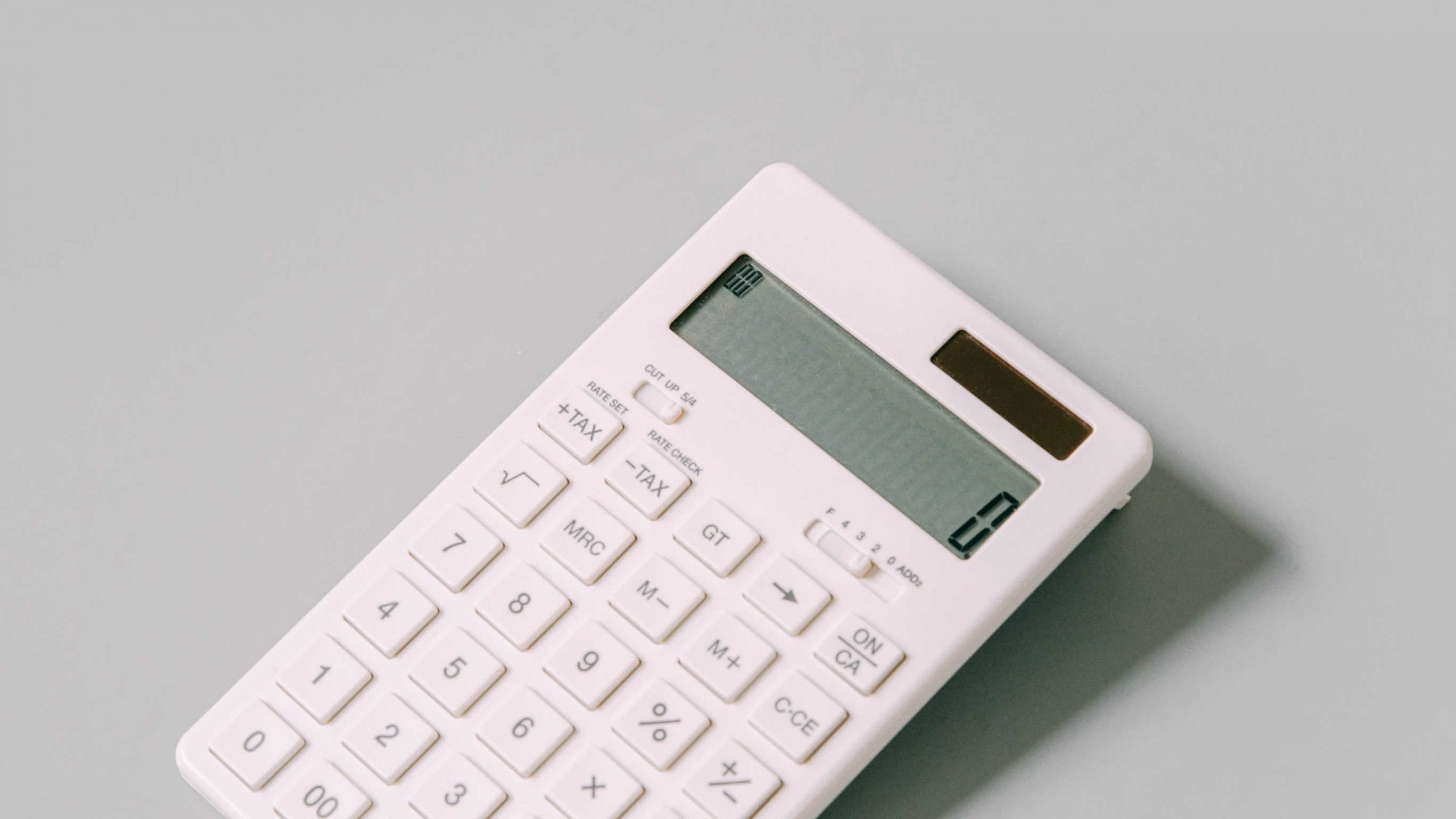
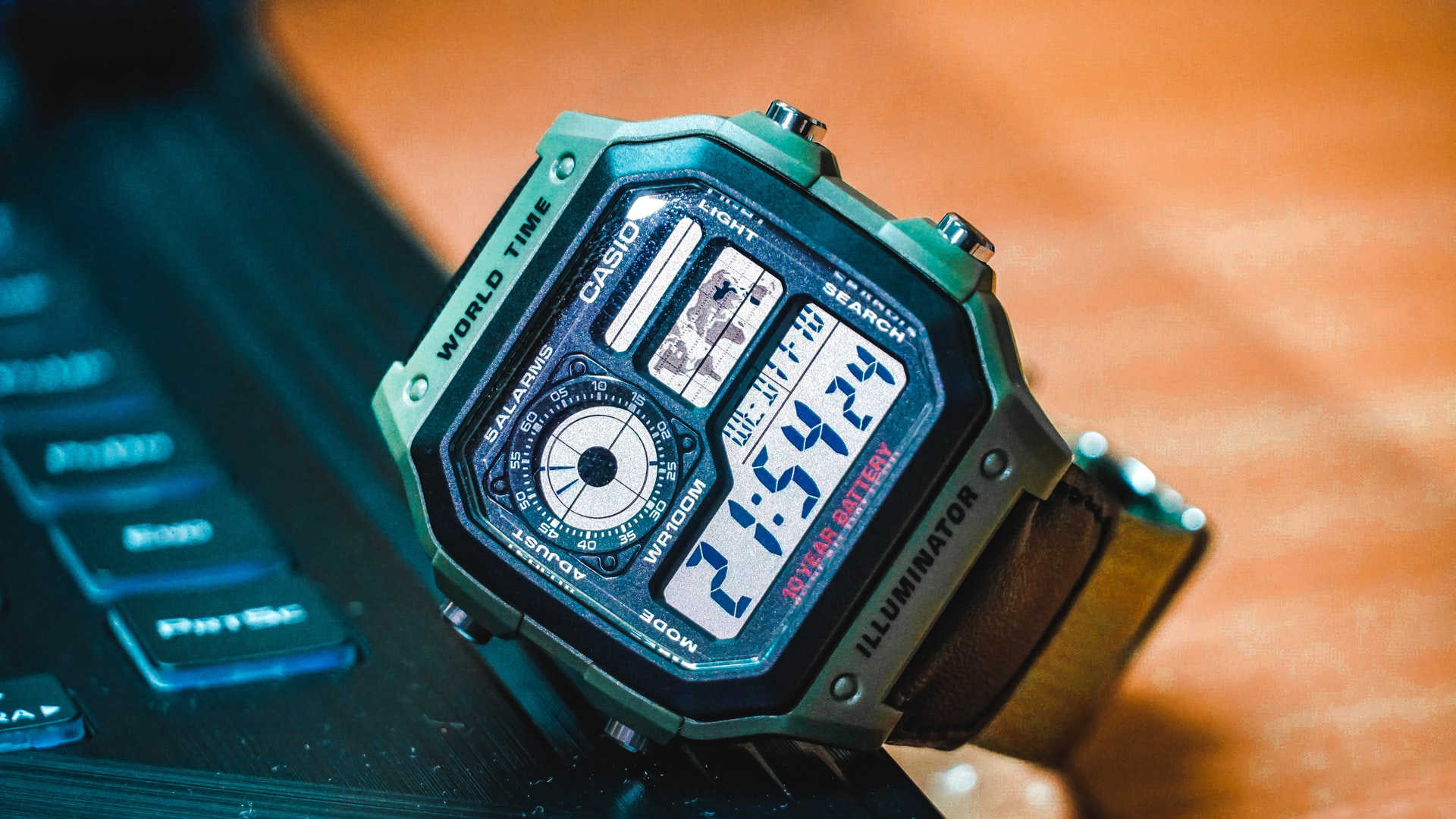
Practice
Try experimenting with the text feature on the Graphic LCD.
- Modify your program to display different text.
- Modify your program so the text is in the bottom right corner. (Hint: modify the values that are 0 to 10 and see what happens).
Check out the advanced lesson Using the Sensor API before you use the API for the first time.